Discover Top Tips for Developing a Cross Platform App: Streamline your build with effective frameworks, ensure seamless UX/UI, and prioritize code reusability for efficient app performance across devices.
Cross platform app development has become increasingly popular in recent years, as businesses strive to reach the largest possible audience.
Developing an app that can run on multiple platforms, including iOS, Android, and Windows, has many advantages, including cost savings, wider market reach, and increased efficiency.
However, creating a cross platform app can be a challenging task, requiring expertise in different programming languages, platforms, and devices.
Key Takeaways:
- Cross platform app development is a popular way to reach a wider audience.
- Developing a successful cross platform app requires expertise in different platforms and devices.
Choose the Right Cross Platform Framework
Cross platform app development requires selecting the appropriate framework that can support the app’s development process across multiple operating systems. Choosing the right framework is crucial for efficient and effective app development. In this section, we will discuss different cross platform frameworks and their pros and cons to help you in selecting the most suitable one for your project.
Types of Cross Platform Frameworks
There are different types of cross platform frameworks available to choose from, including:
Type of Framework | Pros | Cons |
---|---|---|
React Native | – High performance – Large community support – Easy to learn | – Limited access to device features – Requires knowledge of JavaScript |
Xamarin | – Native performance – Large community support – Access to device features | – Limited free resources – Requires knowledge of C# |
Flutter | – Fast development – High-performance – Access to device features | – Limited community support – Requires knowledge of Dart |
Note: The above table is for informational purposes only. It is essential to conduct in-depth research and analysis before selecting a cross platform framework.
Factors to Consider When Choosing a Framework
When selecting a cross platform framework, it is crucial to consider factors such as:
- Compatibility with the target operating systems
- Availability of community support
- The level of access to device features required by the app
- The ease of learning and working with the framework
- The cost of using the framework
Code Example
Below is an example of using React Native to create a basic cross platform app:
import React, { Component } from 'react'; import { Text, View } from 'react-native'; class App extends Component { render() { return ( <View> <Text>Hello, world!</Text> </View> ); } } export default App;
This code creates a simple app that displays “Hello, world!” on the screen. The code is written in JavaScript, which is used in React Native framework.
By considering the factors mentioned above and selecting the most appropriate cross platform framework for your project, you can ensure efficient and effective app development.
Plan for Compatibility Across Operating Systems
Developing an app that is compatible with different operating systems is crucial to its success. Ignoring compatibility can result in a subpar user experience, negative reviews, and ultimately, a loss of revenue. To ensure optimal compatibility, consider the following tips:
1. Research Compatibility Guidelines
Before beginning development, research each operating system’s compatibility guidelines and requirements. These guidelines will outline the necessary features and design elements required to ensure compatibility with the operating system.
2. Use Cross-Platform Tools
Using cross-platform development tools can simplify the process of creating an app that is compatible with multiple operating systems. These tools allow developers to write code once and deploy it across multiple platforms, streamlining the development process and reducing the likelihood of compatibility issues.
3. Test Across Multiple Devices
To ensure compatibility, test the app across multiple devices with different screen sizes, resolutions, and operating systems. This will help identify any compatibility issues and allow for necessary adjustments to be made.
4. Utilize Responsive Design
Implementing responsive design ensures that the app’s user interface adapts to different screen sizes and resolutions. This is essential for ensuring a seamless user experience across different devices.
5. Keep Up With Updates
Stay up to date with the latest operating system updates and ensure the app is compatible with them. Failing to update the app can result in compatibility issues and a loss of users.
By following these tips, you can ensure that your app is compatible with different operating systems, ultimately resulting in a positive user experience and increased revenue.
Optimize User Interface for Multiple Devices
In today’s world, where users access apps on a variety of devices with different screen sizes and resolutions, optimizing the user interface (UI) for multiple devices is critical. Failure to do so can result in a suboptimal user experience, which can negatively impact the success of your app.
Here are some app development tips to optimize your app’s UI for multiple devices:
- Design for the smallest screen size: To ensure that your app is usable on smaller devices, such as smartphones, design your UI for the smallest screen size first. This will force you to focus on the most important elements and prioritize them.
- Create responsive layouts: Responsive layouts automatically adapt to the screen size of the device, providing an optimal viewing experience. Use CSS media queries to create responsive layouts, and test them on different devices to ensure that they work as intended.
- Use flexible images: Images can be a challenge when it comes to optimizing UI for multiple devices. To solve this, use flexible images that can scale without losing their quality. Use vector images wherever possible.
- Streamline navigation: Navigation should be simple and easy to use across different devices. Avoid complex menus and use clear labels to help users find what they are looking for quickly.
- Standardize font sizes: Font sizes should be consistent across different devices. Use a standard font size for body text and headings to avoid inconsistencies.
Code Example:
/* CSS Media queries for responsive layouts */ @media (min-width: 768px) { /* Styles for tablets */ } @media (min-width: 1024px) { /* Styles for desktops */ }
By optimizing your app’s UI for multiple devices, you can ensure a positive user experience and increase the likelihood of your app’s success. Paying attention to these app development tips will enhance your coding skills and level up your cross platform app development game!
Write Modular and Reusable Code
Efficient cross-platform app development requires writing modular and reusable code. By breaking down complex tasks into smaller, more manageable modules, developers can avoid repetition, minimize errors, and improve overall code quality.
Modular code is code that can be reused across different sections of an app and adapted to suit different needs. To write modular code, it is important to identify common functions or processes that occur throughout the app and separate them into discrete, reusable modules.
Reusable code is code that can be used across multiple apps or projects. To make code reusable, it is important to write code that is flexible enough to be adapted to different contexts. This means using generic variable names, providing clear instructions on how to use the code effectively, and minimizing dependencies on other code.
When writing modular and reusable code, it is important to follow best practices for efficient coding. This includes using proper naming conventions, commenting code to explain its purpose, and adhering to a consistent coding style.
Consider the following code example:
//This function takes two numbers as input and returns their sum function calculateSum(num1, num2) { return num1 + num2; } //This function takes an array of numbers as input and returns their total sum function calculateTotal(arr) { let total = 0; for(let i = 0; i < arr.length; i++) { total += arr[i]; } return total; }
In this example, the “calculateSum” function could be reused throughout the app to calculate the sum of different sets of numbers, while the “calculateTotal” function could be reused in other apps or projects that require calculating the total sum of an array of numbers.
By writing modular and reusable code, developers can save time and improve the overall quality of their apps.
Implement Continuous Integration and Testing
Continuous integration (CI) and testing are critical components of cross platform app development. By automating the testing process, developers can identify and fix issues early on, saving time and resources in the long run.
CI involves regularly merging code changes into a shared repository and running automated tests to ensure the app remains functional. This process helps to catch any problems early on, reducing the risk of bugs and errors in the codebase.
Utilizing tools like Jenkins, Travis CI, and CircleCI can help streamline the CI process, making it easier to manage and maintain. These tools can be integrated into continuous deployment pipelines, allowing developers to deploy updates seamlessly.
Testing is an essential aspect of cross platform app development. By testing the app on different devices and operating systems, developers can ensure the app functions correctly on different platforms. Tools like Appium, Selenium, and TestComplete can help automate testing, making it easier to test the app on different platforms.
Example:
Step | Action |
---|---|
Step 1 | Write unit and integration tests for each feature |
Step 2 | Turn on continuous integration for the code repository |
Step 3 | Automate testing using tools like Appium or TestComplete |
Step 4 | Set up a continuous deployment pipeline to automatically deploy updates to the app stores |
By implementing CI and testing processes, developers can ensure the app remains stable and functional across different platforms, providing a seamless user experience.
Optimize Performance and Loading Speed
One of the key challenges in cross platform app development is optimizing performance and loading speed. Users expect apps to be quick and responsive, regardless of the platform they are using. Here are some app development tips to help ensure your app performs at its best:
1. Use a Lightweight Framework
Choosing the right cross platform framework is crucial for optimizing app performance. Look for a lightweight framework that minimizes the amount of code your app needs to run. This can help reduce load times and improve overall performance.
2. Minimize HTTP Requests
Reduce the number of HTTP requests your app makes to improve loading speed. This can be achieved by combining multiple resources, such as CSS and JavaScript files, into a single file. Additionally, consider using a content delivery network (CDN) to speed up resource delivery.
3. Enable Caching
Caching can significantly improve app performance by reducing server requests. Enable caching on all resources, including images and videos. This will allow the app to load faster for repeat users, as the app will store previously accessed resources locally.
4. Optimize Images and Video
Large image and video files can slow down app performance. Optimize all media files to reduce their file size without sacrificing quality. This can be achieved by compressing images and using video formats that are optimized for mobile devices.
5. Minimize JavaScript and CSS
Write efficient JavaScript and CSS code to minimize app size and improve loading speed. Avoid repetitive or unnecessary code, and use minification tools to reduce file size.
6. Implement Lazy Loading
Lazy loading is a technique where content is loaded only when it is needed. This can significantly improve app performance by reducing the amount of content that needs to be loaded at once. Consider using lazy loading for images, videos, and other non-critical content.
7. Test and Optimize Performance
Finally, regularly test and optimize your app’s performance. Use tools like Google’s PageSpeed Insights to identify areas for improvement, and implement best practices for performance optimization on an ongoing basis.
Data Security and Privacy
One of the most crucial aspects of cross platform app development is ensuring data security and privacy. With sensitive user information being shared across multiple platforms, it is essential to take necessary precautions to protect it from unauthorized access or theft.
Implementing secure authentication processes, data encryption, and secure online storage is critical to safeguarding user data. Utilizing third-party libraries to handle sensitive information such as credit card details or personal identification is also recommended to reduce the likelihood of data breaches.
Regularly testing and auditing the app’s security measures can help identify vulnerabilities and areas for improvement. Additionally, ensuring compliance with relevant legal and regulatory requirements is essential in safeguarding user privacy.
Example code:
// Ensure password is hashed before storing in database bcrypt.hash(password, saltRounds, function(err, hash) { // Store hashed password in database }); // Implement secure authentication process passport.use(new LocalStrategy({ usernameField: 'email', passwordField: 'password' }, function(email, password, done) { User.findOne({ email: email }, function (err, user) { if (err) { return done(err); } if (!user) { return done(null, false); } // Compare password hash with stored hash bcrypt.compare(password, user.password, function(err, result) { if (result === true) { return done(null, user); } else { return done(null, false); } }); }); }));
Implementing these security measures can provide peace of mind to users and protect the reputation of your app and business.
Utilize Platform-Specific Features
Utilizing platform-specific features is a powerful technique to enhance the user experience of cross-platform apps. By leveraging the unique capabilities of each operating system, developers can create apps that feel native to each platform while sharing the same codebase.
For example, iOS has a variety of platform-specific APIs, such as Apple Pay and Core ML, that can be used to provide enhanced functionality to users. On the other hand, Android offers features like Material Design and Google Maps integration that can greatly improve the user experience.
To take advantage of these platform-specific features, developers must have a deep understanding of each operating system and its capabilities. It’s essential to research and test which features will work best for each platform and incorporate them seamlessly into the app’s design and functionality.
However, it’s also important to keep in mind that utilizing platform-specific features can add complexity to the app development process. Developers must ensure proper testing and compatibility across various devices and operating systems.
By strategically utilizing platform-specific features, developers can create apps that feel tailored to each platform while maximizing code reuse and development efficiency.
Regularly Update and Maintain the App
Regularly updating and maintaining your cross platform app is essential for ensuring its continued success. In this section, we’ll provide some expert tips for effective app updates and maintenance.
1. Stay up-to-date with the latest operating systems
Operating systems are constantly evolving, and it’s important to stay up-to-date with the latest changes to ensure compatibility with new devices. Be sure to test your app on the latest versions of operating systems and make any necessary updates to ensure optimal performance.
2. Fix bugs and security vulnerabilities
Regularly testing your app for bugs and security vulnerabilities is critical for maintaining its integrity and protecting user data. Ensure that all reported bugs are fixed promptly and that any identified security vulnerabilities are addressed as quickly as possible.
3. Respond to user feedback
Listening to user feedback is an excellent way to improve your app and address any issues that users may be experiencing. Be sure to respond to user comments and reviews, and take their feedback into account when making updates to your app.
4. Optimize app performance
Optimizing your app’s performance is essential for providing a seamless user experience. Conduct regular performance testing to identify any areas where the app can be optimized, and make any necessary updates to improve loading speed and overall performance.
5. Regularly backup data
Regularly backing up user data is critical for ensuring that their data is not lost in the event of a system failure or other issue. Implement a regular backup schedule to ensure that all user data is protected and easily recoverable if needed.
Code Example:
function updateApp() { | // code to update app goes here | } |
---|---|---|
function fixBugs() { | // code to fix bugs goes here | } |
function optimizePerformance() { | // code to optimize performance goes here | } |
By regularly updating and maintaining your cross platform app, you can ensure that it continues to provide a valuable and enjoyable user experience. Follow these tips to ensure that your app stays competitive and relevant in today’s fast-paced tech environment.
Collaborate with Experienced Cross Platform Developers
Developing a cross platform app requires specialized skills that not every developer possesses. Collaborating with experienced cross platform developers can provide a wealth of knowledge and expertise to ensure that your app is optimized for seamless performance on various platforms.
Hiring a dedicated team of cross platform developers can also save time and resources, as they bring a wealth of experience and knowledge to the table. With outsourced development work, businesses can focus on other core activities while leaving cross-platform app development in the hands of experts.
Collaborating with experienced developers also provides an excellent opportunity to learn from their coding skills and working methods. Experienced developers can help educate businesses on the best practices in cross platform app development, and provide advice on the latest trends and technologies.
Furthermore, experienced developers can help businesses stay up-to-date with the latest developments in the field, ensuring that their apps remain relevant and competitive. This can help businesses remain ahead of the curve in the rapidly-evolving world of cross-platform app development.
Overall, collaborating with experienced cross platform developers enables businesses to develop high-quality, optimized apps that perform seamlessly on multiple platforms while saving time and resources, and learning from industry experts.
Conclusion – Top Tips for Developing a Cross Platform App
Developing a cross platform app requires a unique set of skills and strategies to ensure seamless performance across different operating systems and devices. By following these 10 expert tips, app developers can enhance their coding skills and create high-quality multi-platform applications.
Choosing the right cross platform framework, planning for compatibility across operating systems, optimizing user interface for multiple devices, writing modular and reusable code, implementing continuous integration and testing, optimizing performance and loading speed, ensuring data security and privacy, utilizing platform-specific features, regularly updating and maintaining the app, and collaborating with experienced cross platform developers are essential steps in the development process.
External Resources
https://dotnet.microsoft.com/en-us/apps/xamarin
FAQ – Top Tips for Developing a Cross Platform App
Q: What is cross platform app development?
A: Cross platform app development refers to the process of creating mobile applications that can run on multiple operating systems, such as iOS and Android, using a single codebase.
Q: Why is cross platform app development important?
A: Cross platform app development allows developers to reach a wider audience by creating apps that can be used on different devices and platforms. It also reduces development time and costs.
Q: What are the benefits of using a cross platform framework?
A: Using a cross platform framework simplifies the development process by providing tools and libraries that can be used across different platforms. It allows for code reuse and faster development.
Q: How can I ensure compatibility across different operating systems?
A: To ensure compatibility across different operating systems, it is important to test your app on various devices and operating system versions. You can also use platform-specific APIs and libraries to handle compatibility issues.
Q: How can I optimize my app’s user interface for multiple devices?
A: To optimize your app’s user interface for multiple devices, you can use responsive design techniques, such as fluid layouts and adaptive design, which allow the interface to adapt to different screen sizes and resolutions.
Q: What is modular and reusable code?
A: Modular and reusable code is code that is divided into small, self-contained modules that can be reused in different parts of the app or in different apps altogether. This promotes code efficiency and maintainability.
Q: How can continuous integration and testing benefit cross platform app development?
A: Continuous integration and testing help identify and fix bugs and issues early in the development process. This ensures that the app is stable and reliable across different platforms.
Q: How can I optimize my app’s performance and loading speed?
A: To optimize app performance and loading speed, you can use techniques such as code optimization, caching, and lazy loading. It is also important to test your app’s performance on different devices and operating systems.
Q: How can I ensure data security and privacy in my app?
A: To ensure data security and privacy, you should implement secure authentication mechanisms, encrypt sensitive data, and follow best practices for data handling and storage. Regular security audits should also be conducted.
Q: What are platform-specific features and how can I utilize them?
A: Platform-specific features are features that are unique to a particular operating system. You can utilize these features by using platform-specific APIs and libraries to enhance the user experience and take advantage of the capabilities offered by each platform.
Q: Why is it important to regularly update and maintain the app?
A: Regularly updating and maintaining the app is important to ensure compatibility with new operating systems, fix bugs and security vulnerabilities, and provide a seamless user experience. It also shows users that the app is actively supported and improved.
Q: Why should I collaborate with experienced cross platform developers?
A: Collaborating with experienced cross platform developers brings expertise and knowledge to the development process. Experienced developers can help optimize the app, provide guidance on best practices, and ensure a high-quality end product.
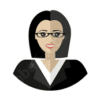
Charlotte Williams is a talented technical author specializing in cross-platform app development. With a diverse professional background, she has gained valuable experience at renowned companies such as Alibaba and Accenture. Charlotte’s journey in the tech industry began as a mobile UX designer back in 2007, allowing her to develop a keen understanding of user-centric app design.
Proficient in utilizing frameworks like React Native and Flutter, Charlotte excels in building cross-platform mobile apps and imparting her knowledge to aspiring developers. She pursued a degree in Computer Science at Cornell University, equipping her with a strong foundation in the field. Residing in San Francisco with her three beloved dogs, she finds solace in hiking the hills and connecting with nature. Charlotte’s passion for app development, combined with her dedication to sharing expertise, makes her an invaluable resource in the world of cross-platform app development.