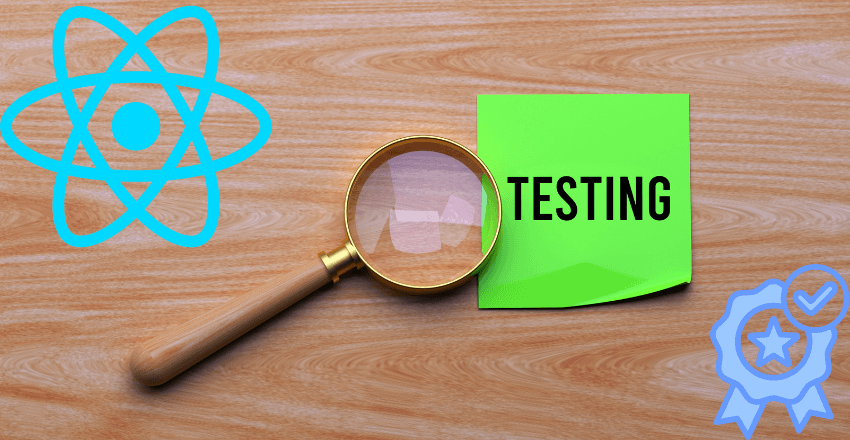
React Native Testing Best Practices streamline development, combining automated testing frameworks with manual testing to catch bugs early and improve code quality.
Importance of Testing in React Native Apps
In the world of mobile app development, testing is a crucial step to ensure the quality and reliability of applications. React Native, a popular framework for building cross-platform mobile apps, is no exception. Testing React Native apps not only helps identify and fix bugs but also allows developers to validate the functionality and usability of their applications.
When it comes to testing React Native apps, developers have access to various testing tools that streamline the testing process and enhance efficiency. These tools provide a range of features that simplify the task of testing, making it easier to identify issues and ensure consistent performance across different platforms.
One of the key testing strategies for React Native apps is unit testing. Unit testing involves testing individual units or components of the application in isolation. This approach helps identify defects at an early stage and enables developers to focus on specific functionalities, making it easier to catch and fix bugs before they impact the overall performance of the app.
In addition to unit testing, integration testing is another essential aspect of testing React Native apps. Integration testing involves testing the interaction of multiple components and ensuring that they work seamlessly together. This type of testing helps identify any integration issues and ensures that the different parts of the app function correctly as a whole.
By leveraging unit testing and integration testing, developers can gain confidence in the quality and stability of their React Native apps. These testing strategies help reduce the risk of bugs and ensure that the app performs as intended on various devices and platforms.

The Benefits of Unit Testing and Integration Testing in React Native Apps
Unit testing and integration testing offer several benefits when it comes to testing React Native apps:
- Improved code quality:Unit testing allows developers to identify and fix bugs early in the development process, resulting in cleaner and more maintainable code.
- Faster development cycles: With automated unit testing and integration testing, developers can catch bugs quickly and make changes with confidence, reducing development time.
- Enhanced app performance: Testing individual units and the integration of components ensures that the app functions correctly and delivers optimal performance.
- Better user experience:Through thorough testing, developers can ensure that the app meets user expectations and functions flawlessly across different devices and platforms.
- Cost savings:By identifying and fixing bugs early, developers can avoid costly issues that may arise later in the app’s lifecycle.
Achieving Comprehensive Test Coverage in React Native
In order to ensure the quality and reliability of React Native applications, it is crucial to achieve comprehensive test coverage. This means thoroughly testing all aspects and functionalities of the app to identify and fix any potential issues or bugs.
One of the key strategies for achieving comprehensive test coverage is throughautomated testing for React Native applications. By automating the testing process, developers can save time and effort while ensuring thorough testing of the app.
There are several tools available for automated testing in React Native. These tools provide a framework for creating and running automated tests, allowing developers to simulate user interactions and test different scenarios. Some popular tools for automated testing in React Native include Jest, Detox, and Appium.
Automated testing for React Native apps offers several benefits. It allows developers to easily repeat tests and ensure consistent results, reducing the risk of human error. Additionally, automated tests can be run across different devices and platforms, ensuring compatibility and a seamless user experience.
When implementing automated testing, it is important to consider the different types of tests that can be conducted. These include unit tests, integration tests, and end-to-end tests. Unit tests focus on testing individual components of the app in isolation, while integration tests ensure that different components work together seamlessly. End-to-end tests simulate real user interactions and test the entire app flow.
By combining different types of automated tests, developers can achieve comprehensive coverage and minimize the need for manual testing. This not only saves time and effort but also improves the overall quality of the React Native app.
Best Practices for React Native Unit Testing
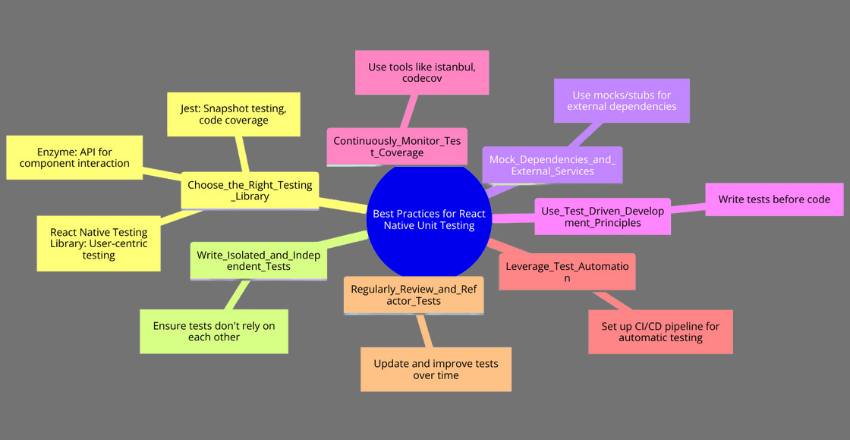
Unit testing is a critical aspect of developing high-quality React Native applications. By testing individual units of code, such as functions and components, we can ensure their correctness and identify any potential issues early in the development process. In this section, I will share some best practices for unit testing in React Native, along with recommended testing libraries and valuable tips.
1. Choose the Right Testing Library
When it comes to unit testing in React Native, selecting the right testing library is essential. Some popular options include:
- Jest: Jest is a widely-used testing library that comes preconfigured with React Native. It offers features such as snapshot testing and code coverage reporting.
- React Native Testing Library: This library provides a user-centric approach to testing React Native components. It focuses on simulating user interactions and asserting the expected component behavior.
- Enzyme: Enzyme is a powerful testing utility for React Native. It offers a convenient API for interacting with React components and asserting their properties and behavior.
2. Write Isolated and Independent Tests
When writing unit tests, it’s vital to ensure that each test is isolated and independent. This means that tests should not rely on the state or behavior of other tests. Isolated tests are easier to understand, maintain, and debug, allowing for more efficient development.
3. Mock Dependencies and External Services
Unit tests should focus solely on the unit of code being tested, while mocking any external dependencies or services. By using mocks or stubs, we can isolate the unit of code and test it in isolation, without relying on the behavior of other components or external systems. This improves test reliability and speed.
4. Use Test-Driven Development (TDD) Principles
Test-driven development (TDD) is a development methodology where tests are written before the code is implemented. By following TDD principles, developers can ensure that the code meets the desired behavior defined by the tests. This approach promotes better code coverage and helps identify potential issues early in the development cycle.
5. Continuously Monitor Test Coverage
Regularly monitoring test coverage is crucial for maintaining the quality of a React Native application. By ensuring that all relevant code paths are covered by tests, we can minimize the risk of undetected bugs. There are several tools available, such as istanbul and codecov, that provide detailed reports on the test coverage of your codebase.
6. Leverage Test Automation
Automation is key to efficient and effective unit testing in React Native. Setting up a CI/CD pipeline that runs tests automatically on code changes can help catch issues early and provide quick feedback to developers. Tools like Jenkins and CircleCI can be integrated with your version control system to automate the testing process.
7. Regularly Review and Refactor Tests
Just like production code, unit tests require regular review and refactoring. As the application evolves, tests may become outdated or less efficient. It’s important to periodically review the tests, update them to reflect code changes, and refactor them to make them more maintainable and readable.
Best Practices for React Native Unit Testing | Benefits |
---|---|
Choose the Right Testing Library | Ensures efficient and reliable testing |
Write Isolated and Independent Tests | Increases test clarity and maintainability |
Mock Dependencies and External Services | Allows for focused unit testing without external factors |
Use Test-Driven Development (TDD) Principles | Improves code coverage and identifies issues early |
Continuously Monitor Test Coverage | Reduces the risk of undetected bugs |
Leverage Test Automation | Enhances efficiency and provides quick feedback |
Regularly Review and Refactor Tests | Maintains test reliability and readability |
By following these best practices, developers can establish a solid foundation for unit testing in React Native. Effective unit testing leads to enhanced code quality, reduced bug count, and improved overall app performance.
Integration Testing Strategies for React Native Apps
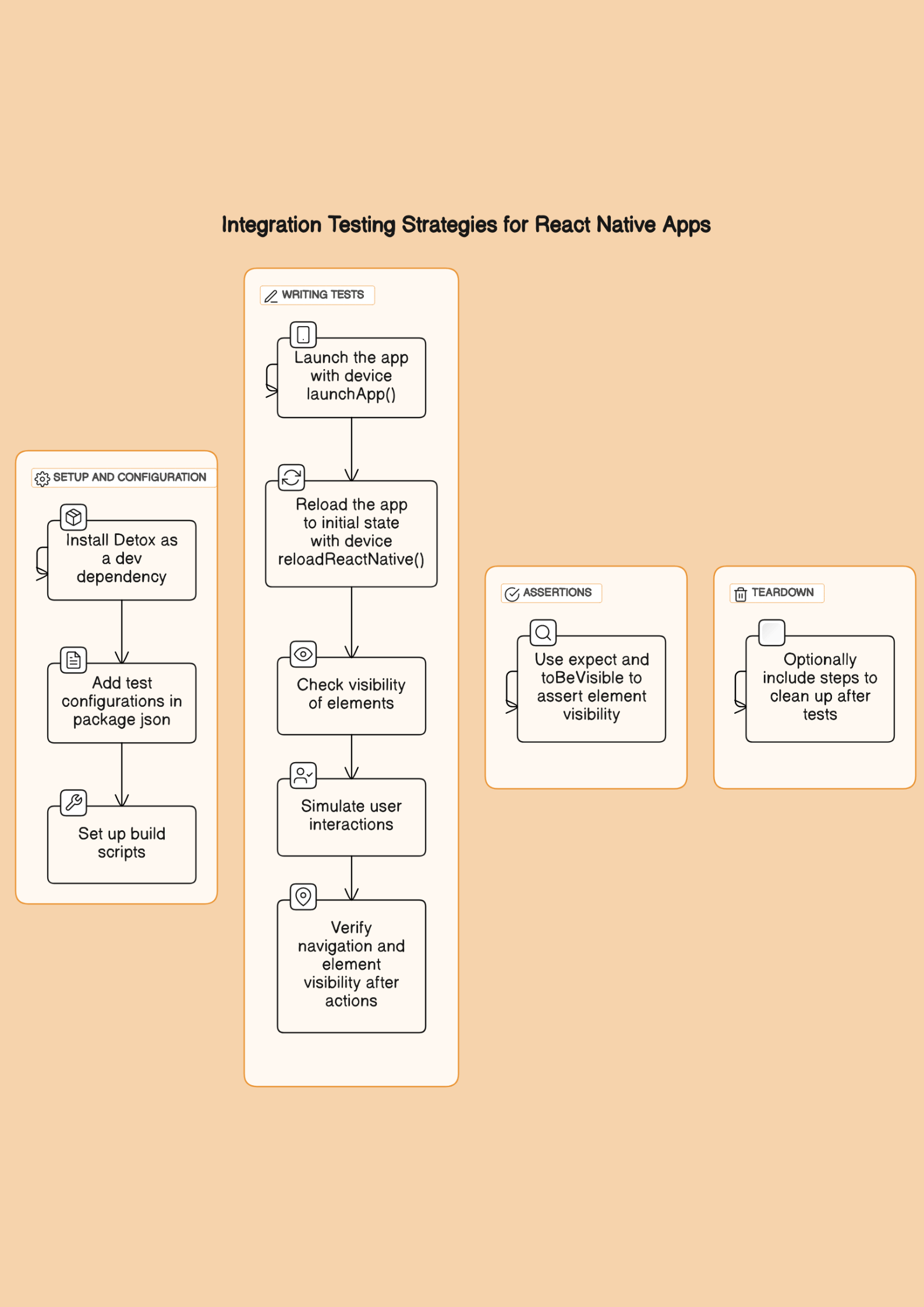
In the realm of React Native app development, integration testing plays a crucial role in ensuring the functionality and reliability of the final product. By simulating real-life user interactions and testing component interactions, integration testing helps identify and mitigate issues that may arise from the integration of various components or external dependencies.
End-to-end testing, a subset of integration testing, focuses on testing the complete flow of an application, ensuring that all the integrated components work seamlessly together. This type of testing provides valuable insights into how the app performs in real-world scenarios.
When it comes to integration testing in React Native, there are several testing libraries available that offer a range of features and capabilities. These testing libraries help developers streamline the integration testing process, providing an efficient and effective way to ensure the stability and quality of their apps.
Code Sample
For integration testing strategies in React Native apps, Detox is a popular and powerful tool that enables end-to-end testing and simulates a user environment. Detox tests aim to mimic user behavior and interactions within the app to validate its flows from start to finish. Below is a code sample demonstrating how to set up and write an integration test using Detox for a simple login flow in a React Native application.
Pre-requisites
Before implementing the test, ensure you have Detox installed and configured for your React Native project. This includes installing Detox as a dev dependency, adding test configurations in your package.json
, and setting up the necessary build scripts. For detailed setup instructions, refer to the Detox documentation.
Integration Test Code Sample: Testing a Login Flow
describe('Login flow integration test', () => {
beforeAll(async () => {
await device.launchApp();
});
beforeEach(async () => {
await device.reloadReactNative();
});
it('should display the login screen', async () => {
await expect(element(by.id('loginScreen'))).toBeVisible();
});
it('should allow a user to enter credentials and login', async () => {
// Assuming there are TextInput components for username and password with testIDs 'usernameInput' and 'passwordInput'
await element(by.id('usernameInput')).typeText('testuser');
await element(by.id('passwordInput')).typeText('password');
// Assuming there's a Button component for submitting the login form with testID 'submitButton'
await element(by.id('submitButton')).tap();
// Assuming success navigates to a 'homeScreen', verify navigation
await expect(element(by.id('homeScreen'))).toBeVisible();
});
// Add more tests as needed to cover the complete login flow
});
Explanation
- Setup and Teardown: The
beforeAll
function is used to launch the app before the test suite runs, andbeforeEach
ensures the app is reloaded to its initial state before each test. - Visibility Check: The first test ensures that the login screen is visible upon app launch, validating the initial app state.
- Interaction and Navigation: The second test simulates a user entering their username and password into the respective fields and then tapping the submit button. It demonstrates how to use
typeText
to simulate typing andtap
to simulate button presses. Finally, it verifies that the app navigates to the home screen upon successful login. - Assertions: The
expect
andtoBeVisible
methods are used to assert that specific elements appear on the screen at the right times, ensuring that the app behaves as expected during the test scenarios.
This code sample provides a basic framework for implementing integration tests in React Native apps using Detox. By adapting and expanding upon these tests, developers can cover a wide range of user interactions and app flows, significantly enhancing the app’s reliability and user experience.
Popular React Native Testing Libraries for Integration Testing
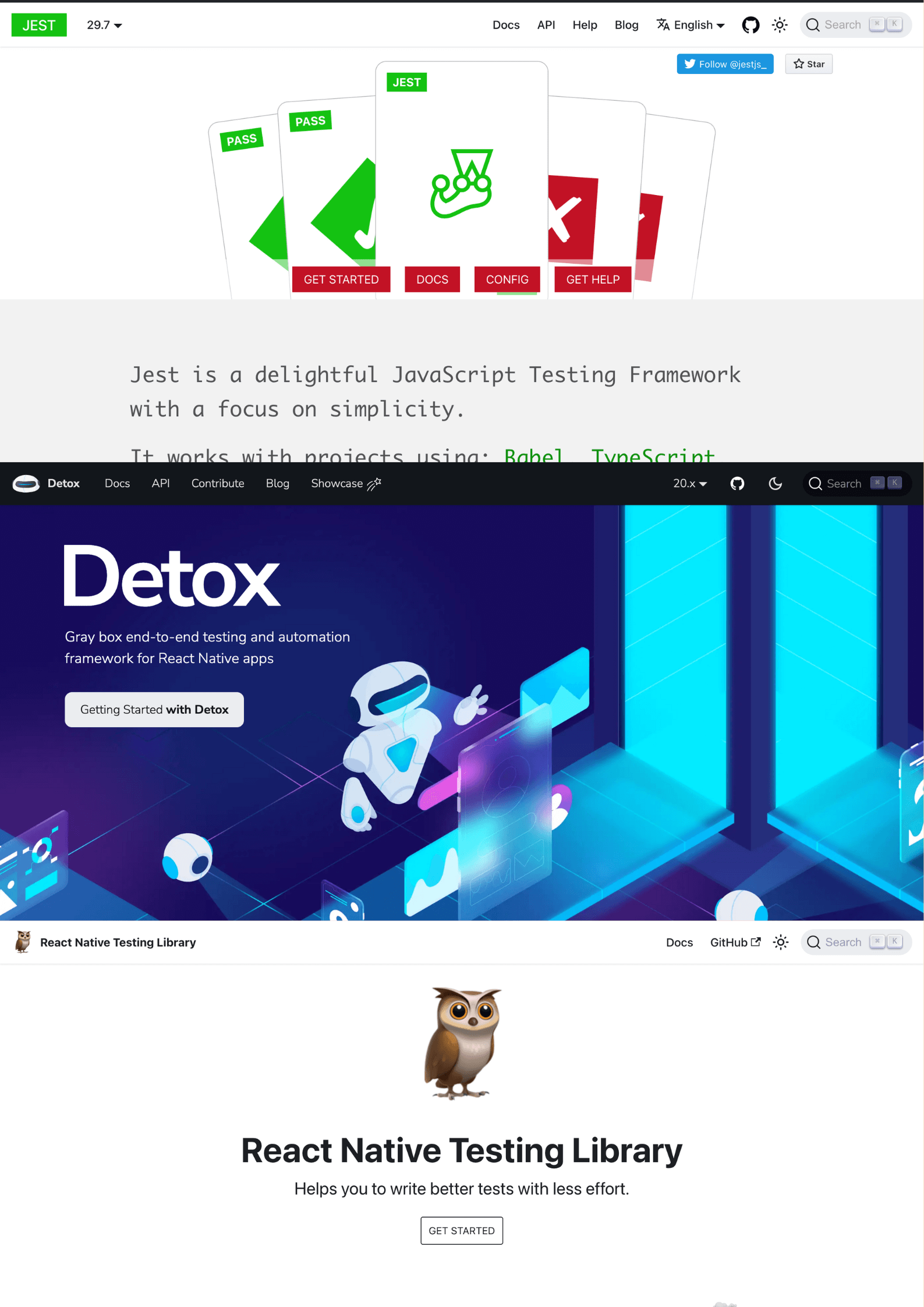
- Jest: Jest is a widely used testing framework that provides powerful tools for integration testing in React Native. With its built-in snapshot testing and mocking capabilities, Jest enables developers to easily write robust integration tests.
- Detox: Detox is a popular end-to-end testing framework specifically designed for React Native apps. It allows developers to write automated tests that simulate user interactions across multiple screens and verify the app’s behavior.
- React Native Testing Library: The React Native Testing Library is a lightweight testing utility that encourages developers to write tests focused on the user’s perspective. It provides a simple API for querying and interacting with React Native components, making it easier to test the integration of components.
By leveraging these testing libraries, developers can effectively test the integration of components, ensuring that the app functions correctly in different scenarios and environments. These libraries provide features and APIs that simplify the testing process, saving time and effort while maintaining the quality of the app.
Wrapping up
Integration testing plays a vital role in ensuring the seamless functioning of React Native apps. Through end-to-end testing, developers can validate the interactions between different components, dependencies, and APIs. By utilizing popular testing libraries, developers can streamline the integration testing process and enhance the overall app stability.
External Resources
https://enzymejs.github.io/enzyme/
https://callstack.github.io/react-native-testing-library/
FAQ
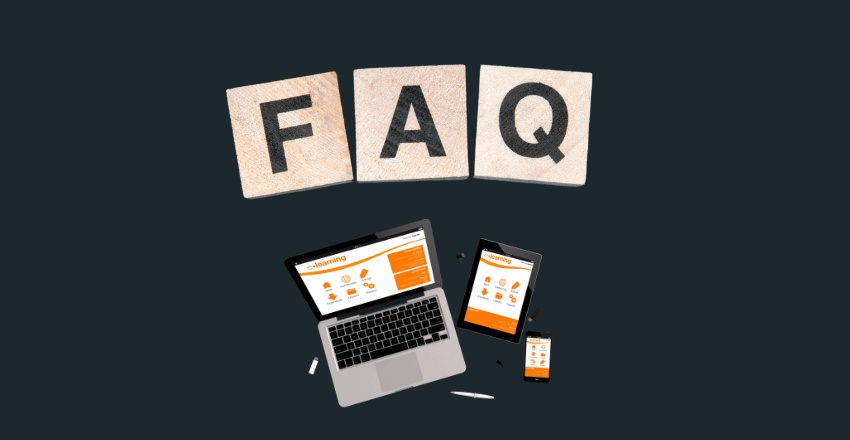
1. How do I write unit tests for React Native components?
Question: How do I write unit tests for React Native components?
Answer:
Unit testing in React Native can be efficiently handled with Jest, a popular testing framework. For testing components, you can use the react-test-renderer
library to render components to pure JavaScript objects without relying on the native environment.
Code Sample:
// Import dependencies
import React from 'react';
import { Text } from 'react-native';
import renderer from 'react-test-renderer';
// Example component to test
const Greeting = ({ name }) => <Text>Hello, {name}!</Text>;
// Unit test for the Greeting component
test('Greeting renders correctly', () => {
const tree = renderer.create(<Greeting name="React Native" />).toJSON();
expect(tree).toMatchSnapshot();
});
Explanation:
This example demonstrates how to test a simple React Native component using Jest and react-test-renderer
. The Greeting
component is tested to ensure it renders the expected output when provided with a name
prop. The toMatchSnapshot
method is used to create a snapshot of the component output, which can be used to detect changes in future tests.
2. How do I perform integration testing in React Native?
Question: How do I perform integration testing in React Native?
Answer:
Integration testing in React Native can be accomplished with the Detox framework. Detox provides a gray-box testing solution that allows you to simulate user interactions and test the integration of multiple components within your app.
Code Sample:
First, install Detox and configure it for your project according to the official documentation.
Then, write an integration test:
describe('User login flow', () => {
beforeAll(async () => {
await device.reloadReactNative();
});
it('should login the user', async () => {
await expect(element(by.id('loginScreen'))).toBeVisible();
await element(by.id('emailInput')).typeText('user@example.com');
await element(by.id('passwordInput')).typeText('password');
await element(by.id('loginButton')).tap();
await expect(element(by.id('welcomeScreen'))).toBeVisible();
});
});
Explanation:
This Detox test simulates a user logging into the app. It starts by reloading the React Native environment, then proceeds to type an email and password into the respective input fields and taps the login button. Finally, it verifies that the welcome screen is visible after the login action, indicating a successful integration test of the login flow.
3. How can I test React Native components with user interactions?
Question: How can I test React Native components with user interactions?
Answer:
Testing components with user interactions in React Native can be effectively done using the @testing-library/react-native. This library provides a set of helpers to simulate user actions and verify component behavior.
import React from 'react';
import { fireEvent, render } from '@testing-library/react-native';
import Button from '../Button'; // Import your button component
test('button press calls onPress prop', () => {
const onPressMock = jest.fn();
const { getByText } = render(<Button onPress={onPressMock} title="Press Me" />);
fireEvent.press(getByText('Press Me'));
expect(onPressMock).toHaveBeenCalled();
});
Explanation:
This test uses @testing-library/react-native
to render a Button
component and simulate a press event on it. It verifies that the onPress
prop function is called when the button is pressed. This approach is useful for testing how components react to user interactions, ensuring they respond as expected.

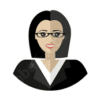
Charlotte Williams is a talented technical author specializing in cross-platform app development. With a diverse professional background, she has gained valuable experience at renowned companies such as Alibaba and Accenture. Charlotte’s journey in the tech industry began as a mobile UX designer back in 2007, allowing her to develop a keen understanding of user-centric app design.
Proficient in utilizing frameworks like React Native and Flutter, Charlotte excels in building cross-platform mobile apps and imparting her knowledge to aspiring developers. She pursued a degree in Computer Science at Cornell University, equipping her with a strong foundation in the field. Residing in San Francisco with her three beloved dogs, she finds solace in hiking the hills and connecting with nature. Charlotte’s passion for app development, combined with her dedication to sharing expertise, makes her an invaluable resource in the world of cross-platform app development.