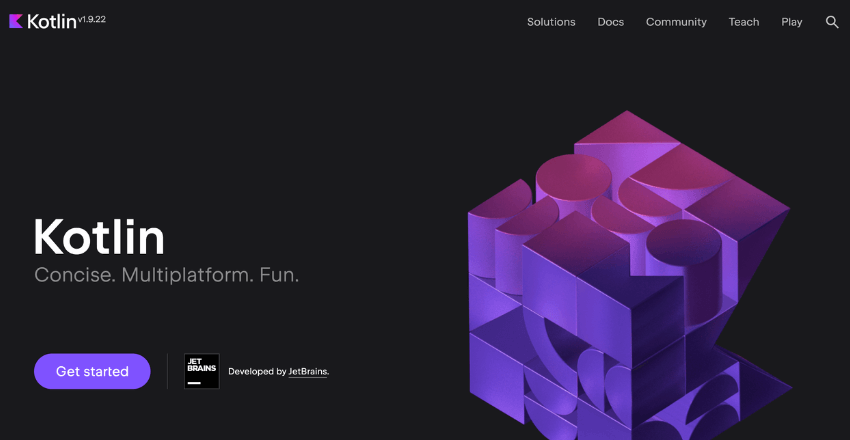
As a Kotlin developer, understanding design patterns and best practices is crucial to enhancing coding efficiency and software quality. Kotlin is an open-source, modern language that blends the best of object-oriented and functional programming paradigms.
By following effective Kotlin practices, developers can create maintainable, scalable and efficient code.
Key Takeaways | Details |
---|---|
Understanding Kotlin Design Patterns | Kotlin’s concise syntax and blend of object-oriented and functional programming paradigms support the implementation of various design patterns, simplifying code maintenance and improving scalability. |
Singleton Design Pattern | Kotlin simplifies the Singleton pattern implementation through the “object” keyword, ensuring a class has only one instance with global access, lazy initialization, and thread safety. |
Builder Design Pattern | The Builder pattern in Kotlin helps avoid constructor overload by providing a fluent interface for setting object properties, leading to immutable objects ideal for multithreaded environments. |
Factory Design Pattern | The Factory pattern in Kotlin promotes loose coupling by allowing object creation without specifying concrete classes, enhancing maintainability and scalability. |
Observer Design Pattern | Kotlin’s Observer pattern facilitates a one-to-many relationship between objects, enabling automatic notification of state changes to observers, which promotes flexibility and scalability. |
Decorator Design Pattern | The Decorator pattern in Kotlin allows for dynamic addition of behaviors to objects at runtime without altering their original classes, enhancing flexibility and maintainability. |
Best Practices for Kotlin Development | Key practices include efficiently organizing code, proper use of nullable types, maintaining concise syntax, leveraging Kotlin standard library functions, and continuous learning and application of design patterns. |
Continuous Learning and Collaboration | Mastering Kotlin design patterns is an ongoing process that involves continuous learning, applying patterns in real-world projects, and collaborating with fellow developers to share insights and experiences. |
Benefits of Design Patterns | Design patterns in Kotlin offer numerous benefits, including simplified code maintenance, improved scalability, enhanced readability, and a structured approach to software development challenges. |
Implementation Examples | Examples provided for Singleton, Builder, Factory, Observer, and Decorator patterns demonstrate how to apply these patterns in Kotlin, highlighting their benefits and practical uses. |
Mastering Design Patterns | Mastering Kotlin design patterns is crucial for writing maintainable, scalable, and efficient code, leading to higher software quality and productivity in Kotlin development projects. |
This table summarizes the key points from the article, emphasizing the importance of understanding and applying design patterns in Kotlin for enhanced coding efficiency, software quality, and maintainability.
Kotlin Design Patterns
Kotlin is becoming an ever popular choice among developers due to its concise and expressive syntax, its interoperability with Java, and the ease with which it allows building robust applications. Design patterns are essential in software development as they provide proven solutions to commonly occurring problems. Kotlin supports the implementation of various design patterns, which can simplify code maintenance and improve scalability.
Kotlin design patterns are the templates or guidelines that developers can follow to create reusable solutions to common software development challenges. These patterns provide a structured approach to problem-solving which can be leveraged to achieve efficient coding. Kotlin design patterns are effective in enhancing coding efficiency, software quality, and maintainability.
Using design patterns in Kotlin development helps to promote code that is easy to read, modify, and extend. Standardizing the best development practices ensures that any developer who joins the project later can quickly understand the code structure and purpose. In addition, it reduces the chances of introducing bugs or making mistakes.
What Are Design Patterns?
Design patterns are reusable solutions to commonly recurring problems in software development. They provide a structured approach to solving these problems and have been proven to be effective over time. Design patterns provide a standard way of representing solutions to specific problems, making it easier to communicate coding challenges among developers.
A design pattern is not a finished code; rather, it’s an agreed-upon approach to solving a problem in software development. A design pattern includes a description of the problem, the solution, and the advantages and disadvantages of the said solution. Implementing a design pattern involves customizing it to meet specific requirements and making it work with the rest of the codebase.
Design patterns have become popular in software development because they help to make code more maintainable and extensible. They can help to simplify coding while reducing the potential for bugs and errors.

Singleton Design Pattern in Kotlin
Kotlin provides an elegant way to implement the Singleton design pattern, which ensures that a class has only one instance that can be accessed globally. The Singleton pattern is useful in scenarios where you need to control the access and creation of a shared resource, such as a database connection or a logging mechanism.
To create a Singleton class in Kotlin, you can use the “object” keyword instead of the traditional class keyword. The “object” declaration creates a class with a single instance that is lazily initialized upon first access. Here is an example:
// Singleton class in Kotlin
object Logger {
fun log(message: String) {
println(message)
}
}
// usage:
Logger.log("Hello World!")
The above code declares a Singleton class called “Logger” with a single function “log” that prints a message to the console. To use the Logger class, you can call its “log” function as shown in the commented line at the end of the code snippet.
The Singleton pattern offers several benefits, including:
- Global access: The Singleton object can be accessed from anywhere in the code, making it easy to share resources and ensure consistency among multiple components.
- Lazy initialization: The Singleton instance is created only when it is first accessed, which can save memory and improve performance.
- Thread safety: The Singleton instance is inherently thread-safe since it is created only once and accessed by multiple threads.
The Singleton pattern can be used in many scenarios, such as managing a database connection, creating a cache, or implementing a logger.
Builder Design Pattern in Kotlin

The Builder design pattern is a creational pattern that provides a flexible solution for creating complex objects. With this pattern, you can separate the construction of an object from its representation, allowing you to vary its internal representation. The resulting object is immutable, making it ideal for multithreaded environments.
When used in Kotlin, the Builder design pattern helps to avoid constructor overload. Instead of a constructor with many parameters, the Builder pattern provides a fluent interface to configure the object properties.
Let’s take a look at an example:
“Imagine you have a class called Person with several properties, including name, age, gender, and profession. Using the traditional approach, you would create a constructor that takes all of these properties as parameters. This approach can quickly become confusing and difficult to use when you have many properties. With the Builder pattern, you can separate the construction of the class from its representation.”
Here’s the code example:
class Person private constructor(
val name: String,
val age: Int,
val gender: String,
val profession: String
) {
data class Builder(
var name: String = "",
var age: Int = 0,
var gender: String = "",
var profession: String = ""
) {
fun name(name: String) = apply { this.name = name }
fun age(age: Int) = apply { this.age = age }
fun gender(gender: String) = apply { this.gender = gender }
fun profession(profession: String) = apply { this.profession = profession }
fun build() = Person(name, age, gender, profession)
}
}
// Usage example
val person = Person.Builder()
.name("John Doe")
.age(30)
.gender("Male")
.profession("Software Engineer")
.build()
- Use of
apply
: The Builder class methods now use theapply
scope function. This allows for thethis
context to be implicit inside the block, making the code more concise and idiomatic. - Method Chaining: By returning the Builder instance (
this
) from each setter method, we enable fluent API style, allowing for method chaining which is a common pattern in Kotlin for setting properties. - Data Class (Optional): While not strictly necessary for the Builder pattern, converting the Builder into a data class could provide additional benefits like
toString
,equals
, andhashCode
implementations for free, which can be useful for debugging or logging. However, in the context of a Builder class designed solely for building another class’s instances, this might not always be beneficial. It’s included here for completeness but can be omitted based on your use case.
These changes result in cleaner, more idiomatic Kotlin code that leverages Kotlin’s features for more readable and concise implementation of the Builder pattern.
In this example, we have a Person class with four properties. We also have a nested Builder class, which has setters for each property and a build() method to create the Person object. In the main function, we create a new Person object using the Builder pattern.
The benefits of using the Builder pattern in Kotlin include:
- Reduced constructor overload
- Easy and readable configuration of object properties
- Immutable objects, making them safe for multithreaded environments
By using the Builder design pattern in Kotlin, you can simplify the creation of complex objects and improve the readability of your code.
Factory Design Pattern in Kotlin
Kotlin’s Factory design pattern is a creational pattern that allows for creating objects without specifying their concrete classes. The Factory pattern defines an interface for creating objects, but lets subclasses decide which objects to instantiate.
Using the Factory pattern in Kotlin can enhance code maintainability by decoupling the creation of objects from the code that uses them. This reduces the impact of changes to the way objects are created, making it easier to modify class hierarchies without affecting client code.
Implementing the Factory Pattern in Kotlin
The following code example demonstrates how to implement the Factory pattern in Kotlin:
sealed interface Animal {
fun makeSound()
}
class Dog : Animal {
override fun makeSound() = println("Woof!")
}
class Cat : Animal {
override fun makeSound() = println("Meow!")
}
object AnimalFactory {
fun createAnimal(type: String): Animal? = when (type) {
"Dog" -> Dog()
"Cat" -> Cat()
else -> null
}
}
- Sealed Interface: Using a sealed interface (or class) for
Animal
limits the types of animals that can be created, enhancing when expression exhaustiveness checks. This means if more animal types are added in the future, the compiler will enforce handling these new types in thewhen
expression, reducing the risk of errors. - Singleton Factory: The
AnimalFactory
class is converted to anobject
declaration, making it a singleton. This is a common pattern for factories in Kotlin, as it doesn’t require multiple instances of the factory to create animals. - Expression Bodies: Simplified
makeSound
implementations inDog
andCat
classes to use expression bodies, which are more concise and idiomatic for simple methods that return a single expression. - Type Safety and Readability: The use of a sealed interface and concise syntax makes the code more readable and safer, as it is clear which types can be created and how they are handled.
The Animal interface defines a makeSound method that must be implemented by its subclasses, Dog and Cat. The AnimalFactory class defines a createAnimal method that takes a string parameter and returns a new instance of the appropriate subclass.
For example, to create a new Dog object, the following code can be used:
val dog = AnimalFactory.createAnimal("Dog")
dog?.makeSound() // Output: Woof!
Given that AnimalFactory
has been converted to an object
(a singleton), you no longer need to instantiate it with AnimalFactory()
. Instead, you directly call the createAnimal
method on AnimalFactory
. This approach is more concise and takes full advantage of Kotlin’s object declaration for implementing singleton patterns.
Thoughts:
- Direct Access: By making
AnimalFactory
anobject
, you can accesscreateAnimal
directly on theAnimalFactory
without needing to instantiate it. - Safe Call Operator (
?.
): The safe call operator is used to callmakeSound
only ifdog
is not null, which is a succinct way to handle the method call on a potentially null object returned bycreateAnimal
. - Graceful Null Handling: This pattern allows for graceful handling of unrecognized types by returning
null
fromcreateAnimal
and then safely handling the potential null object with the safe call operator.
This cleaner approach leverages Kotlin’s language features for more straightforward and idiomatic code, particularly in handling singletons and nullable types.
The createAnimal method returns null if the type parameter is not recognized, allowing for graceful error handling when creating objects.

Benefits of Using the Factory Pattern in Kotlin
The Factory pattern promotes loose coupling between classes, making it easier to modify class hierarchies without impacting client code. It allows for dynamic object creation at runtime, enabling more flexible and maintainable code.
The Factory pattern is particularly useful in scenarios where object creation is complex or requires conditional logic. By separating object creation from the rest of the code, the Factory pattern can simplify code maintenance and improve scalability.
Observer Design Pattern in Kotlin
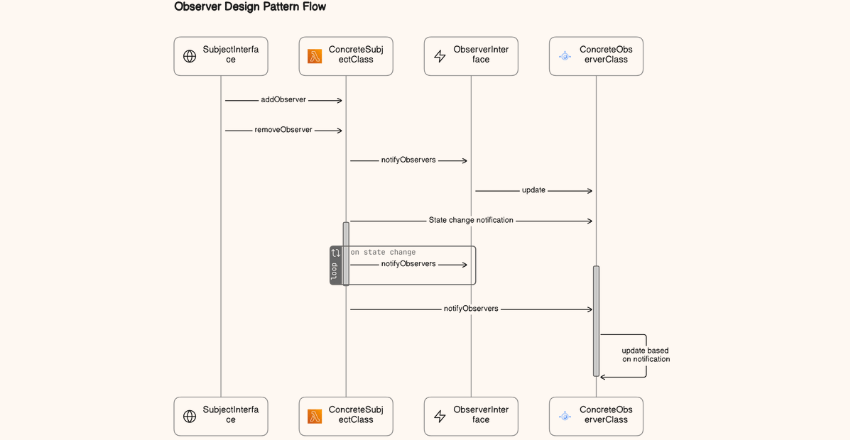
The Observer design pattern is a popular Kotlin design pattern that establishes a one-to-many relationship between objects. In this pattern, an object (known as the subject) maintains a list of its dependents (known as observers) and notifies them automatically of any state changes.
The Observer pattern is useful in achieving maintainable and extensible code and is commonly used in user interfaces, event-driven systems, and notification systems. It enables loose coupling between objects and promotes flexibility and scalability.
Implementing the Observer Design Pattern in Kotlin
In Kotlin, the Observer pattern can be implemented using interfaces, classes, and lambdas.
First, we define the Subject interface that holds the list of observers and has methods for adding and removing observers:
```
interface Subject {
var observers: MutableList
fun addObserver(observer: Observer)
fun removeObserver(observer: Observer)
fun notifyObservers()
}
```
Next, we define the Observer interface that requires an update method to receive notifications from the subject:
```
interface Observer {
fun update()
}
```
Finally, we implement the ConcreteSubject class that implements the Subject interface and manages the list of observers:
```
class ConcreteSubject: Subject {
override var observers: MutableList = mutableListOf()
override fun addObserver(observer: Observer) {
observers.add(observer)
}
override fun removeObserver(observer: Observer) {
observers.remove(observer)
}
override fun notifyObservers() {
observers.forEach { it.update() }
}
}
```
We can then implement the ConcreteObserver class that implements the Observer interface and updates its state based on notifications from the subject.
For example, let’s say we have a concrete observer class called “BatteryDisplay” that displays the battery level of a mobile device. We can implement it as follows:
```
class BatteryDisplay(private val subject: Subject): Observer {
private var batteryLevel: Int = 0
init {
subject.addObserver(this)
}
override fun update() {
batteryLevel = getBatteryLevel()
display()
}
private fun getBatteryLevel(): Int {
// code to obtain battery level
}
private fun display() {
// code to display battery level
}
}
```
Here, the BatteryDisplay class receives updates from the subject and updates its batteryLevel instance variable accordingly. It then displays the battery level using the display method.
Advantages of the Observer Design Pattern in Kotlin
The Observer design pattern in Kotlin offers several advantages, including:
- Loose coupling between objects, enabling flexibility and scalability
- Maintaining modular and extensible code
- Enabling event-driven programming
By using the Observer pattern, we can create more efficient, maintainable, and scalable code in Kotlin.
Decorator Design Pattern in Kotlin
The Decorator design pattern is a structural pattern that allows you to dynamically add behaviors to an object at runtime by wrapping it with a decorator object. This pattern is useful for extending the functionality of an object without modifying its original implementation.
In Kotlin, the decorator pattern can be implemented using class inheritance or composition. The decorator object wraps the original object and provides additional functionality by delegating calls to the wrapped object and performing additional operations as necessary.
Here’s an example of implementing the Decorator pattern in Kotlin:
interface Widget {
fun draw()
}
class TextField : Widget {
override fun draw() = println("Drawing a text field")
}
// Extension function to add border functionality dynamically
fun Widget.withBorder(): Widget = object : Widget {
override fun draw() {
this@withBorder.draw() // Draw the original widget
println("Drawing a border around the widget") // Then draw the border
}
}
fun main() {
val textFieldWithBorder = TextField().withBorder()
textFieldWithBorder.draw()
}
Thoughts:
- Extension Functions: By using an extension function (
withBorder
), we can dynamically add behavior to anyWidget
without needing a separate decorator class. This makes the pattern more flexible and reusable across differentWidget
implementations. - Anonymous Class: Inside the
withBorder
extension function, an anonymous class implementingWidget
is used to wrap the original widget and add the border-drawing functionality. This technique allows for a concise and clear implementation of the decorator functionality without a named class. - Delegated Call: The
draw
method of the anonymous class first delegates the call to the original widget’sdraw
method (usingthis@withBorder.draw()
) and then adds its behavior (drawing a border). - Simplified Main Function: The main function demonstrates how to apply the decorator to a
TextField
object in a straightforward and readable manner, showcasing the pattern’s practical application.
In this example, the interface Widget represents the original object, and the class TextField is the concrete implementation. The BorderDecorator class wraps the TextField object and adds a border around it by calling the draw() function on the wrapped object and then drawing the border.
The Decorator pattern is useful when you want to add functionality to an object at runtime, without affecting other instances of the same class. It also allows for better organization of code by separating responsibilities into small, focused classes.
By using the Decorator pattern, you can achieve more flexible and maintainable code in your Kotlin development projects.
Best Practices for Kotlin Development
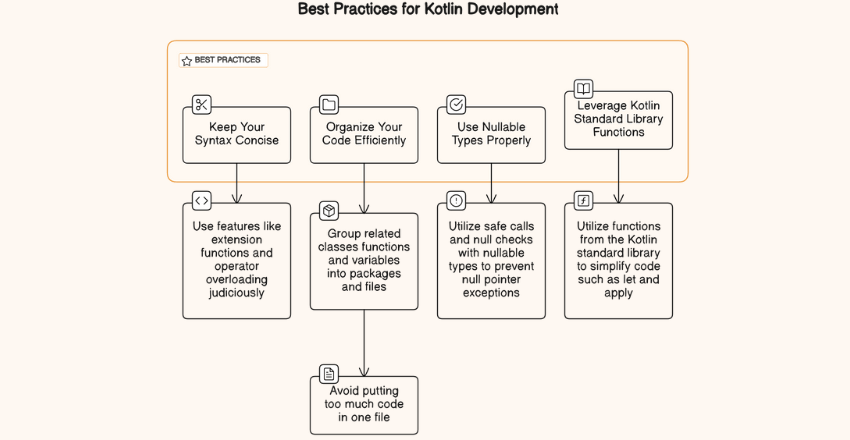
Kotlin is a powerful language that simplifies coding and increases productivity. However, to leverage its full potential, you need to follow some best practices that will help you write better, more efficient code. In this section, we will share some essential best practices for Kotlin development that will help you write maintainable and extensible code.
Organize Your Code Efficiently
One of the best practices in Kotlin development is to organize your code efficiently. You should group related classes, functions, and variables into packages and files. This will make it easier to navigate your code and find what you need quickly.
You should also avoid putting too much code in one file. If a file becomes too long, it will become difficult to read and maintain. Instead, split it into smaller files, each with a specific purpose.
Use Nullable Types Properly
Kotlin introduced nullable types, which allow you to declare a variable that can hold either a value or null. While nullable types can be beneficial in some cases, they can also lead to null pointer exceptions if not used correctly.
It is essential to use safe calls and null checks when working with nullable types. This will ensure that your code is not affected by unexpected null values.
Keep Your Syntax Concise
Kotlin offers concise syntax that allows you to write code quickly and efficiently. However, overusing some of the language’s features, such as extension functions and operator overloading, can make your code hard to understand.
It is essential to use these features judiciously and keep your code’s syntax concise and readable. This will make it easier for other developers to understand your code and contribute to the project.
Leverage Kotlin Standard Library Functions
Kotlin provides a rich standard library that includes many helpful functions that you can use to simplify your code. For example, you can use the let function to execute a block of code on a non-null object, and the apply function to configure an object’s properties.
By using these functions, you can write cleaner, more efficient code that is easier to read and maintain.
Code Example: Using the Let Function
data class User(val name: String, var age: Int)
// Example of using `apply` for object configuration
val newUser = User("John Doe", 25).apply {
age = 30 // Modifying property without needing to refer to the object explicitly
}
// Example of using `let` for null-safe operations
val nullableUser: User? = User("Jane Doe", 28)
nullableUser?.let {
// This block will only be executed if nullableUser is not null
println("The user's name is ${it.name} and age is ${it.age}")
}
Thoughts:
- Using
apply
: Theapply
function is great for initializing or configuring objects. It allows you to modify the object’s properties or call its methods within the block without explicitly referring to the object itself. This can make your initialization code more concise and readable. - Using
let
for Null Safety: Thelet
function is particularly useful for executing a block of code on a non-null object. It provides a concise and safe way to perform operations on nullable objects without the risk of aNullPointerException
.
These examples demonstrate effective ways to leverage Kotlin’s standard library functions to write cleaner, safer, and more idiomatic Kotlin code.
Mastering Kotlin Design Patterns
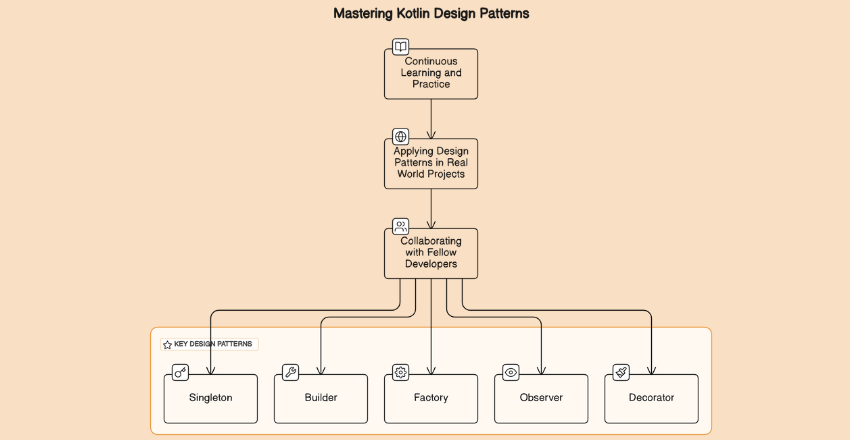
Understanding and implementing Kotlin design patterns is a vital step towards maximizing software quality. The use of design patterns can greatly enhance coding efficiency and extend software scalability. As a cross-platform app development company, we recognize the importance of continually improving our programming skills and knowledge of best practices.
It is important to note that mastering Kotlin design patterns is an ongoing process that requires consistent learning and practice. By employing design patterns, developers can improve code readability, maintainability, and extensibility. Kotlin design patterns, including Singleton, Builder, Factory, Observer, and Decorator, are a few examples of efficient design patterns that developers can implement to enhance software quality.
Continuous Learning and Practice
Learning Kotlin design patterns is not a one-time event, but a continuous process. Developers should invest time in understanding the principles of each design pattern and the benefits they can offer. By continually improving their understanding of design patterns, developers can apply them efficiently to different projects, and utilize them to optimize software quality.
Applying Design Patterns in Real-World Projects
It is one thing to understand the theory of Kotlin design patterns, but applying them in real-world projects is another important step for mastering them. Developers gain practical experience in applying design patterns through hands-on coding experience. Therefore, we encourage developers to take on projects that allow them to apply Kotlin design patterns effectively, enabling them to build skills and confidence in their abilities.
Collaborating with Fellow Developers
Collaborating with other developers is another excellent way to learn and master Kotlin design patterns. Discussions with fellow developers can provide new insights, enhance understanding of topics, and support developers in troubleshooting challenges. At Hire Cross Platform Developer, our developers regularly engage with one another to discuss design patterns and share their experiences with each other to strengthen their understanding and develop their expertise.
Final Thoughts
Kotlin design patterns are crucial to enhance software quality in app development. By understanding and applying these patterns, developers can achieve efficient and robust coding practices and develop maintainable and scalable software. At Hire Cross Platform Developer, our developers continuously improve their understanding and implementation of Kotlin design patterns and leverage them to deliver high-quality cross-platform app development projects.
External Resource
FAQ
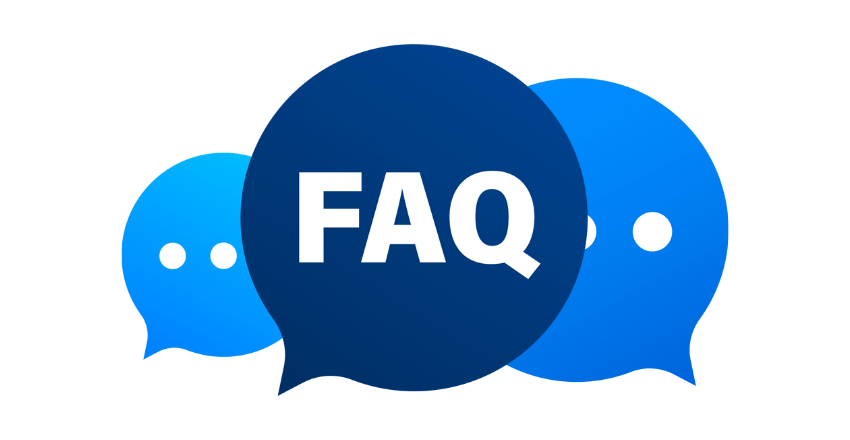
FAQ 1: How can I implement the Singleton pattern effectively in Kotlin?
Answer:
Kotlin provides a straightforward way to implement the Singleton pattern through its object
declaration, which ensures that a class has only one instance throughout the application lifecycle. This is particularly useful for scenarios where you need a single instance, such as for a configuration manager or a logging utility.
Code Sample:
object ConfigurationManager {
var config: Map<String, String> = emptyMap()
fun loadConfig() {
// Simulate loading configuration
config = mapOf("url" to "http://example.com", "timeout" to "5000")
}
}
fun main() {
ConfigurationManager.loadConfig()
println(ConfigurationManager.config)
}
Explanation:
The ConfigurationManager
object is declared using the object
keyword, making it a Singleton. The loadConfig
function simulates loading configuration into a map. Since there’s only one instance of ConfigurationManager
, the configuration is accessible globally in a consistent state.
FAQ 2: How do I use the Builder pattern in Kotlin for complex objects?
Answer:
Kotlin’s named and default arguments partially eliminate the need for the Builder pattern. However, for cases where an object’s construction process is particularly complex or requires additional validation, a more traditional Builder pattern can still be beneficial. Kotlin allows for a clean implementation of this pattern.
Code Sample:
class Pizza private constructor(
val size: String,
val cheese: Boolean,
val pepperoni: Boolean,
val mushrooms: Boolean
) {
class Builder(
private val size: String
) {
private var cheese: Boolean = false
private var pepperoni: Boolean = false
private var mushrooms: Boolean = false
fun cheese(value: Boolean) = apply { cheese = value }
fun pepperoni(value: Boolean) = apply { pepperoni = value }
fun mushrooms(value: Boolean) = apply { mushrooms = value }
fun build() = Pizza(size, cheese, pepperoni, mushrooms)
}
}
fun main() {
val pizza = Pizza.Builder("large")
.cheese(true)
.pepperoni(true)
.build()
println(pizza)
}
Explanation:
The Pizza
class is constructed with a private constructor and a nested Builder
class. The Builder pattern is implemented with fluent methods for each configuration option, returning this
(via apply
) for chaining. The build
method constructs the Pizza
instance.
FAQ 3: What is the best way to implement the Factory pattern in Kotlin?
Answer:
The Factory pattern is useful in Kotlin when you need to create objects without exposing the instantiation logic to the client and refer to newly created objects through a common interface. Kotlin’s concise syntax makes implementing the Factory pattern straightforward and clean.
Code Sample:
sealed class Animal {
abstract fun makeSound(): String
companion object Factory {
fun createAnimal(type: String): Animal = when (type) {
"Dog" -> Dog()
"Cat" -> Cat()
else -> throw IllegalArgumentException("Unknown animal type")
}
}
class Dog : Animal() {
override fun makeSound() = "Woof!"
}
class Cat : Animal() {
override fun makeSound() = "Meow!"
}
}
fun main() {
val dog = Animal.createAnimal("Dog")
println(dog.makeSound())
val cat = Animal.createAnimal("Cat")
println(cat.makeSound())
}
Explanation:
The Animal
sealed class serves as a base for all animal types, ensuring type safety and that all possible subclasses are known at compile time. The Factory
companion object defines the createAnimal
method, which dynamically creates instances of Dog
or Cat
based on the input type. This encapsulates object creation logic within the Animal
class hierarchy, adhering to the Factory pattern principles.

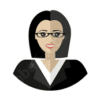
Charlotte Williams is a talented technical author specializing in cross-platform app development. With a diverse professional background, she has gained valuable experience at renowned companies such as Alibaba and Accenture. Charlotte’s journey in the tech industry began as a mobile UX designer back in 2007, allowing her to develop a keen understanding of user-centric app design.
Proficient in utilizing frameworks like React Native and Flutter, Charlotte excels in building cross-platform mobile apps and imparting her knowledge to aspiring developers. She pursued a degree in Computer Science at Cornell University, equipping her with a strong foundation in the field. Residing in San Francisco with her three beloved dogs, she finds solace in hiking the hills and connecting with nature. Charlotte’s passion for app development, combined with her dedication to sharing expertise, makes her an invaluable resource in the world of cross-platform app development.