
Choosing Between Cross Platform and Native Development sets the foundation for delivering exceptional user experiences across diverse device ecosystems efficiently.
One of the biggest decisions when developing a mobile app is choosing the right development approach. Two popular options are cross platform development and native development. Each approach comes with its own set of advantages and limitations, making it essential to choose wisely based on individual app development needs.
Cross Platform Development
Cross platform development is a popular approach to multi-platform app development. This development approach enables creating apps that can run on different platforms utilizing a single codebase. The primary benefit of cross platform development is the ability to develop the app once and deploy it on all platforms. However, cross platform development is not an ideal choice for all app development scenarios.
Comparing both development approaches, cross platform development has less emphasis on platform-specific features and functionalities. Instead, it focuses on creating a unified user interface and a similar user experience across all platforms. While the development process is faster due to code reusability, cross platform development may have some performance limitations. Since cross platform apps depend on a framework, it may not perform as smoothly as native apps.

Exploring Native Development
Native development is an approach to app development that focuses on creating apps for specific platforms using platform-specific languages and tools. This approach offers several advantages, including better performance, greater access to platform-specific features, and a more seamless integration with the platform’s user experience.
However, native development can also be limited by its platform specificity. It requires developers to create and maintain separate codebases for each platform, which can increase development costs and timelines. Additionally, it can be challenging to find skilled developers for each platform, particularly in emerging markets.
Despite these challenges, native development remains a popular choice for many app developers, particularly those looking to create high-performance, feature-rich apps that provide a superior user experience. When deciding between cross platform and native development, it’s essential to consider factors such as the target audience, app complexity, performance requirements, time to market, and budget, in order to make an informed multi-platform decision.
Factors to Consider for Choosing the Right Approach
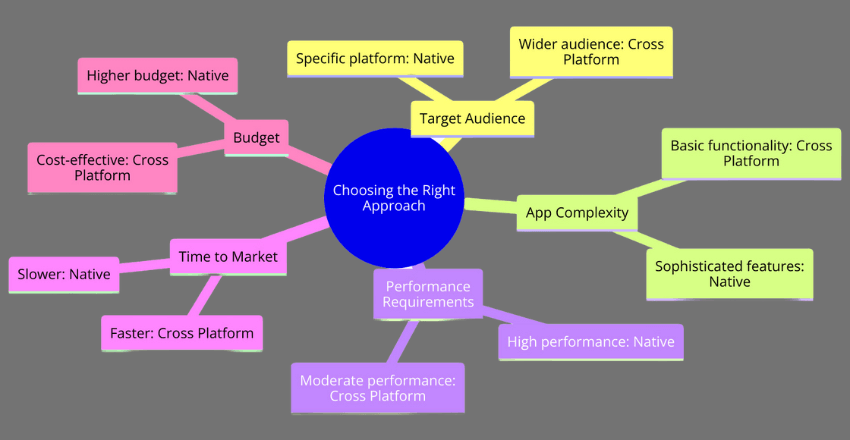
When making the decision between cross platform development and native development, there are several key factors that need to be taken into account. Each approach has its own advantages and disadvantages, and the optimal choice depends on various app development needs and goals. Here are some of the most important factors to consider:
Target Audience
The target audience is a crucial consideration when choosing between cross platform and native development. If the app is intended for a specific platform, such as iOS or Android, native development may be the better option as it can provide a more optimized user experience. On the other hand, if the app needs to cater to a wider audience across multiple platforms, cross platform development can offer the advantage of code reusability and cost-effectiveness.
App Complexity
The complexity of the app is another important factor to consider. Cross platform development may be suitable for simpler apps with basic functionality, while native development is better suited for more complex apps with sophisticated features. This is because native development allows for seamless integration with platform-specific functionalities, while cross platform development may face limitations in accessing certain features.
Performance Requirements
Performance is a critical consideration when developing an app, and it is important to identify the required performance benchmark before choosing a development approach. Native development can offer superior performance, as it utilizes platform-specific languages and tools to optimize performance. Cross platform development, on the other hand, may face challenges related to performance due to the compatibility layer between the app and the platform.
Time to Market
The time to market is another crucial consideration when developing an app. Cross platform development can enable faster time to market, as it allows for the creation of an app that can run on multiple platforms simultaneously. Native development may take longer to develop as it requires separate coding for each platform.
Budget
Budget is a significant consideration when choosing between cross platform and native development. While cross platform development can offer cost savings due to code reusability, native development may require a higher budget due to the need for separate coding for each platform and the requirement for platform-specific tools.
By taking these factors into account, app developers can make an informed decision on whether to choose cross platform development or native development for their app development needs.
Pros and Cons of Cross-Platform Development

Before choosing cross platform development as your development approach, it is important to consider the advantages and disadvantages of this method. While cross platform development may seem like a cost-effective and efficient solution, there are some limitations to this approach.
Pros
- Code reusability: One of the biggest advantages of cross platform development is the ability to reuse the same codebase across multiple platforms. This can save time and reduce development costs.
- Multi-platform support: Cross platform development allows for the creation of apps that work seamlessly across different platforms, including iOS, Android, and web platforms.
- Time to market: With cross platform development, you can launch your app on multiple platforms simultaneously, reducing the time to market.
Cons
- Performance limitations: Cross platform development may face limitations in accessing certain platform-specific features, which may affect the performance of the app as a whole.
- UI limitations: UI elements may not be as polished or user-friendly in cross platform development, as the app has to cater to multiple platforms simultaneously.
- Development limitations: Cross platform development may require developers to rely on third-party frameworks, which may limit their control over the development process.
It is important to weigh the pros and cons of cross platform development before making a decision. Your decision may depend on the specific needs of your app, including your target audience, app complexity, and performance requirements.

Pros and Cons of Native Development
Native development involves the use of platform-specific languages and tools to create apps that are optimized for individual platforms. This approach offers several advantages over cross platform development, but also comes with its own set of challenges.
Pros of Native Development
- Superior Performance: Native apps are faster and more responsive than cross platform apps, as they are optimized for specific platforms and use platform-specific APIs.
- Access to Platform-Specific Features: By using native development, developers can take full advantage of platform-specific features, such as push notifications, widgets, and more. This can lead to a more optimized and personalized user experience.
- Better User Experience: Native development allows for seamless integration with platform-specific user interface elements, resulting in a more cohesive and intuitive app experience for users.
Cons of Native Development
- Higher Development Costs: Since native development involves the use of platform-specific languages and tools, it requires a higher level of expertise and specialized resources. This can lead to higher development costs compared to cross platform development.
- Longer Timeframes: Developing a native app for each platform requires more time and effort, as each app needs to be developed separately and tested on its respective platform.
- Limited Target Audience: An app developed natively for a single platform is only accessible to users on that particular platform, which may limit its reach and potential user base.
In making a multi-platform decision, these pros and cons should be carefully considered alongside the factors outlined in Section 4 to make an informed decision.
Code Examples in Cross Platform Development
In cross platform development, a significant advantage is the ability to write a single codebase that can run on multiple platforms. To illustrate this, let’s take a look at a simple example of creating an app that works on both iOS and Android using a cross-platform framework.
The code snippet below shows how to create a “Hello World” app using Xamarin Forms, a popular cross-platform development tool:
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms" xmlns:x=
"http://schemas.microsoft.com/winfx/2009/xaml" x:Class="HelloWorld.MainPage">
<StackLayout>
<Label Text="Hello World!" VerticalOptions="CenterAndExpand" HorizontalOptions="CenterAndExpand" />
</StackLayout>
</ContentPage>
This code creates a simple “Hello World” app with a label displayed in the center of the screen. With Xamarin Forms, this same code can be used for both iOS and Android platforms, without the need to write separate code for each platform. This is a clear benefit of cross platform development, as it significantly reduces development time and cost.
However, it’s worth noting that not all features and functions are available on all platforms when using cross platform development tools. There may be limitations on accessing native platform-specific features, such as camera, audio, or GPS. This can affect the functionality and performance of the app.
Despite these limitations, cross platform development is an effective approach for many app development projects, particularly those with a limited budget and timeline.
Code Examples in Native Development
Native development relies on platform-specific languages and tools to create apps that are optimized for each platform. This approach often requires developers to have expertise in multiple languages and can result in longer development times and higher costs. However, native development offers advantages such as superior performance and access to platform-specific features.
Let’s take a look at some code examples in native development:
iOS Example: Implementing a Navigation Bar
To implement a navigation bar in iOS, we first need to import the UIKit framework and create a navigation controller:
import UIKit class ViewController:
UIViewController { override func viewDidLoad()
{ super.viewDidLoad() let vc = UIViewController() let navController =
UINavigationController(rootViewController: vc)
navController.navigationBar.prefersLargeTitles = true navController.navigationItem.
title =
"My App Title" self.present(navController, animated: true, completion: nil) } }
In this example, we’re creating a simple view controller and adding it to a navigation controller. We’re also setting the navigation bar to display large titles and setting the title for the navigation item.
Android Example: Creating a Spinner Dropdown
To create a spinner dropdown menu in Android, we first need to create an ArrayAdapter to populate the dropdown options:
ArrayAdapter<String> adapter = new ArrayAdapter<String>
(this, android.R.layout.simple_spinner_item, options);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
spinner.setAdapter(adapter);
In this example, we’re creating an ArrayAdapter with a list of options and setting the layout for the dropdown items. We’re then setting the adapter for the Spinner view.
As you can see, native development requires developers to have expertise in platform-specific languages and tools. While it may take longer to develop apps using this approach, the resulting apps offer superior performance and access to platform-specific features.
Performance Considerations

When choosing between cross platform and native development, one of the most important considerations is app performance. While cross platform development offers the benefit of code reusability and faster development time, it may struggle with performance when compared to native development.
Native development, on the other hand, is optimized for the specific platform it is built for, resulting in superior performance. However, this approach can be more time-consuming and costly, as it requires separate development for each platform.
Factors that affect app performance include app speed, memory usage, and UI responsiveness. Apps built with cross platform development frameworks may face limitations in these areas due to the generic nature of the codebase.
Native development, on the other hand, allows for seamless integration with platform-specific features, resulting in optimized performance. However, this approach requires skilled developers with knowledge of platform-specific programming languages and tools.
Ultimately, the decision between cross platform and native development should be based on the specific performance requirements of the app being developed, as well as the available resources and development timeline.
Platform-Specific Features and User Experience
When it comes to delivering an optimized user experience, platform-specific features play a crucial role. Native development allows for seamless integration with platform-specific functionalities, providing a better user experience. For instance, Android offers customizability options like customizable launchers, while iOS allows for integration with Apple’s Siri digital assistant.
Cross platform development may face limitations in accessing certain platform-specific features, leading to a less optimized user experience. For example, while cross platform development frameworks like React Native and Xamarin allow for the creation of apps that can run on multiple platforms, they may face challenges when accessing certain platform-specific features like user accessibility settings or camera functionalities.
While cross platform development may offer cost-effectiveness and code reusability benefits, it is crucial to weigh the impact of these benefits on the user experience and functionality of the app. Native development allows for better integration with platform-specific features, providing a more optimized experience for the end user.
Development Time and Cost Considerations
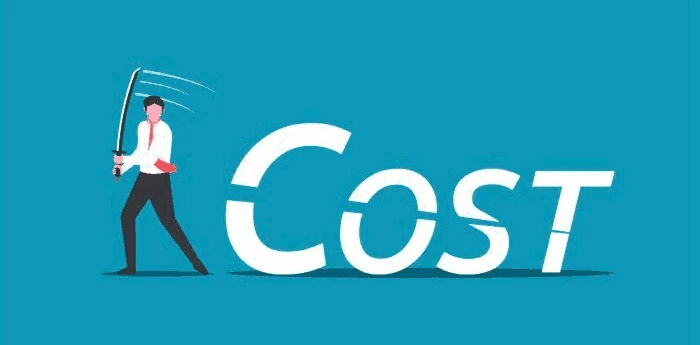
When deciding between cross platform and native development, time and cost are important considerations. Cross platform development allows for the creation of apps that can run on multiple platforms with a single codebase, resulting in cost savings. However, native development involves creating apps for specific platforms using platform-specific languages and tools, making it more costly and time-consuming.
One factor to consider is development timelines. Cross platform development can result in faster development timelines since a single codebase is used, while native development may take longer due to the need to create separate codebases for each platform. However, it should be noted that the quality and functionality of the app should not be compromised for the sake of speed.
Maintenance costs are also a consideration. Cross platform development may result in lower maintenance costs since updates and bug fixes can be implemented for all platforms with a single codebase. On the other hand, native development may require separate updates and bug fixes for each platform, resulting in higher maintenance costs.
Availability of skilled developers is another factor to consider. Cross platform development may have a larger pool of developers since it uses widely available programming languages and frameworks. However, native development requires developers with specific platform expertise.
Factors to Prioritize
Ultimately, the decision between cross platform and native development should be based on the specific needs and goals of the app being developed. Factors such as target audience, app complexity, performance requirements, time to market, and budget should be prioritized. For example, if the app requires access to platform-specific features, native development may be the better option, but if cost-effectiveness and speed of development are top priorities, cross platform development may be the way to go.
Scalability and Future Maintenance
When considering the scalability and future maintenance of an app, choosing the right development approach is crucial. Cross platform development allows for easier updates and maintenance due to the use of a single codebase. However, native development allows for seamless integration with platform-specific features and better performance optimization.
In terms of scalability, cross platform development can be more efficient due to the ability to maintain a single codebase while deploying on multiple platforms. This streamlines the update and maintenance process and reduces the overall cost of ownership. However, if a platform-specific feature is critical to the success of the app, native development may be the better choice, despite the added development and maintenance costs.
Future maintenance is also an important consideration. In a cross platform development environment, updates and bug fixes can be implemented across all platforms simultaneously, ensuring consistency and minimizing the risk of user frustration. Native development, on the other hand, may require more resources and time to implement updates and bug fixes due to the need for separate codebases for each platform.

Examples of Apps Built with Cross Platform and Native Development
When deciding between cross platform and native development, it’s important to consider the success stories of apps built using each approach. Here are two examples showcasing successful apps built using cross platform and native development:
Example 1: Cross Platform Development
The popular app “Untappd” was built using cross platform development. It allows users to discover and rate various types of beer, join communities, and connect with friends. Untappd was able to achieve its multi-platform goals while maintaining a consistent user experience across different platforms using Xamarin, a widely used cross platform framework. According to its co-founder, the app’s codebase was reduced by 70%, and development time was cut in half by using a single codebase for all platforms. Untappd’s success proves that cross platform development can be an effective approach for creating successful apps.
Example 2: Native Development
The widely used app “Instagram” was built using native development. It is a photo and video sharing platform that is available on both iOS and Android platforms. Instagram leverages platform-specific features and functionalities such as camera APIs and push notifications to provide a seamless user experience. The app’s success can be attributed to its superior performance, exceptional user experience, and seamless integration with platform-specific functionalities. Instagram’s success story reinforces the importance of native development in achieving a high-quality user experience.
Making the Right Choice: Factors to Prioritize
Choosing between cross platform development and native development is an important decision that requires careful consideration of multiple factors. Some of the key factors to prioritize are:
- Target audience: Consider the demographics and preferences of your target audience. Cross platform development may be more suitable for reaching a wider audience, while native development allows for a more tailored user experience.
- App complexity: Evaluate the complexity of your app and the need for access to platform-specific features. Native development is better suited for complex apps with high performance requirements.
- Performance requirements: Consider the need for high app speed, memory usage, and UI responsiveness. Native development has a clear advantage in meeting these requirements.
- Time to market: Evaluate the timeline for releasing your app. Cross platform development typically has shorter development timelines due to the reusability of code.
- Budget: Determine the available budget for app development. Cross platform development is typically more cost-effective due to the ability to create one codebase for multiple platforms.
It is important to prioritize these factors based on your unique app development needs and goals. By doing so, you can make an informed decision between cross platform and native development that will lead to the best possible outcome.
Optimal App Development Approach
Choosing the right development approach for an app is crucial to ensure its success. While cross platform development offers benefits such as code reusability and cost-effectiveness, native development focuses on delivering optimized performance and access to platform-specific features.
When making the decision between cross platform and native development, it’s important to consider factors such as the target audience, app complexity, performance requirements, time to market, and budget. This will help determine the development approach that will best meet the needs and goals of the project.
Although cross platform and native development offer different advantages and limitations, they both have a role to play in app development. It’s essential to weigh the pros and cons carefully, and prioritize the factors that are most important for the app’s success.
Overall, there is no one-size-fits-all approach to app development, and the optimal approach will vary depending on the specific requirements of each project. By taking the time to properly evaluate the options and make an informed decision, you can set your app up for success.
About Hire Cross Platform
When it comes to cross platform development, having access to a team of experienced developers is essential. Hire Cross Platform is a company that specializes in providing cross-platform app developers from South America.
Our team is made up of highly skilled developers who have extensive experience in developing cross platform apps across various industries. From e-commerce to gaming, our developers can create apps that work seamlessly across different platforms.
At Hire Cross Platform, we understand the importance of delivering high-quality apps that meet the unique needs of our clients. That’s why we work closely with our clients to ensure that their app development needs are met within their budget and timeline.
Whether you’re looking to develop a new app or need assistance with maintaining an existing one, we’ve got you covered. Our team of cross-platform app developers from South America are dedicated to delivering exceptional results that exceed your expectations.
External Resource
https://blog.iron.io/how-one-developer-serves-millions-of/
FAQ

FAQ 1: What are the key factors to consider when choosing between cross-platform and native app development?
Answer:
When deciding between cross-platform and native app development, several key factors must be considered:
- Performance: Native development generally offers better performance and smoother animations because the code is optimized for a specific platform.
- Development Cost and Time: Cross-platform development can be more cost-effective and faster, as a single codebase can target multiple platforms.
- UI/UX Consistency: Native development allows for a UI/UX that matches platform-specific guidelines more closely, enhancing user experience.
- Feature Access: Native apps can easily access and utilize all device-specific features, while cross-platform apps may have limitations or require additional plugins.
- Maintenance and Updates: Maintaining and updating a cross-platform codebase can be simpler since changes are applied across all platforms at once.
Code Sample: Not applicable as the answer is conceptual and involves strategic decision-making rather than coding.
FAQ 2: How does the choice between cross-platform and native development affect testing strategies?
Answer:
The choice between cross-platform and native development significantly influences your testing strategy due to differences in environment and tools:
- Cross-Platform Testing involves ensuring consistency across multiple platforms from a single codebase. Tools like Appium or Detox can automate UI tests across both iOS and Android.
- Native Testing requires separate tests for iOS and Android, utilizing platform-specific tools such as XCTest for iOS and Espresso for Android for more detailed and precise testing.
Code Sample: Not applicable as the focus is on testing strategy rather than specific code implementation.
FAQ 3: Can you mix cross-platform and native development approaches in a single project? How?
Answer:
Yes, it’s possible to blend cross-platform and native development to leverage the strengths of both approaches. This hybrid strategy can be beneficial for accessing platform-specific features or optimizing performance-critical sections of an app while maintaining overall development efficiency.
Example Approach:
- Use a Cross-Platform Framework like React Native for the majority of the app to ensure quick development across iOS and Android.
- Implement Native Modules for performance-critical features or when needing to access specific native APIs that are not supported or optimally handled by the cross-platform framework.
Code Sample:
// React Native code calling a native module (example for iOS)
import { NativeModules } from 'react-native';
// Assuming a native module 'PerformanceOptimization' exists
const { PerformanceOptimization } = NativeModules;
PerformanceOptimization.optimizeFunctionality()
.then((result) => console.log(result))
.catch((error) => console.error(error));
Explanation:
In this simplified example, a React Native app calls a custom native module (PerformanceOptimization
) to execute some performance-critical operation. This approach demonstrates how a project can primarily use a cross-platform framework while still benefiting from native development for specific needs.

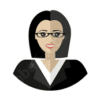
Charlotte Williams is a talented technical author specializing in cross-platform app development. With a diverse professional background, she has gained valuable experience at renowned companies such as Alibaba and Accenture. Charlotte’s journey in the tech industry began as a mobile UX designer back in 2007, allowing her to develop a keen understanding of user-centric app design.
Proficient in utilizing frameworks like React Native and Flutter, Charlotte excels in building cross-platform mobile apps and imparting her knowledge to aspiring developers. She pursued a degree in Computer Science at Cornell University, equipping her with a strong foundation in the field. Residing in San Francisco with her three beloved dogs, she finds solace in hiking the hills and connecting with nature. Charlotte’s passion for app development, combined with her dedication to sharing expertise, makes her an invaluable resource in the world of cross-platform app development.