
Cross platform development has become increasingly popular in recent years, as businesses seek to reach customers on multiple devices and platforms. However, this approach comes with its own unique set of challenges that developers must overcome to create effective and user-friendly applications.
The Cross Platform Landscape
Developing cross platform applications is becoming increasingly popular due to the numerous benefits, including expanded reach and reduced development time. However, this approach presents unique challenges that must be addressed to ensure a successful project.
It is important to understand the cross platform landscape and the particularities of each operating system to overcome development obstacles and create effective solutions. In this section, we will delve into the complexities of cross platform development and discuss the challenges that developers commonly face.
Dealing with Development Obstacles
Cross platform development poses several challenges that must be handled adeptly. Firstly, the compatibility of applications across various devices and systems is critical. Developers must take into account the distinct characteristics of each system, such as screen size, resolution, and hardware functionalities.

Secondly, versioning challenges must be tackled, as each system may have a different software version. Furthermore, UI design and user experience must be carefully planned to ensure consistency and usability across all platforms.
Lastly, cross platform development requires ongoing testing and debugging, which can be challenging due to variations in system features and performance.
To avoid common cross platform development challenges, it is essential to adopt an agile development approach that emphasizes regular communication and collaboration among team members.
Continuous integration and deployment frameworks can also streamline the development process and ensure the timely delivery of consistent and high-quality applications.
By embracing the unique nature of cross platform development, developers can create effective, cutting-edge solutions that engage and delight users across diverse platforms.
Compatibility Issues and Versioning Challenges

Cross platform development poses unique challenges when it comes to ensuring compatibility and maintaining version control. As developers work with different operating systems, software versions, and devices, compatibility issues naturally arise. These challenges can slow down development and create an obstacle to delivering a seamless user experience.
Developers must consider the impact of varying software versions, hardware specifications, and system architecture as they create cross platform applications. Compatibility issues may arise when the application relies on system-level tools or platform-specific features. As a result, it’s essential to ensure that the application functions optimally across a wide range of platforms and devices.
Additionally, as software versions change, it’s necessary to ensure that the application remains compatible with newer versions of the operating system and software. This requires testing the application across various software and hardware configurations, which can be time-consuming and resource-intensive.
To deal with these challenges, developers have several strategies at their disposal. For example, they can use cross platform development frameworks or libraries that offer built-in compatibility and versioning management. Developers can also use automated testing tools that enable them to test the application on multiple devices and platforms simultaneously.
Strategies for Dealing with Compatibility Issues and Versioning Challenges
To overcome compatibility issues and versioning challenges, developers can take several steps:
- Use cross-platform development frameworks: Frameworks like React Native and Flutter are designed to be cross-platform compatible and automatically manage versioning and compatibility. These frameworks provide developers with powerful tools for creating applications that work seamlessly across multiple platforms.
- Implement version control: Developers must use a version control system to manage changes to the application codebase. This ensures that the application can be rolled back to a previous version in case of compatibility issues with newer versions of software.
- Conduct rigorous compatibility testing: Developers must test the application rigorously across different software versions, hardware configurations, and operating systems. This requires a variety of devices and testing tools to ensure proper functionality.
- Use automated testing tools: Automated testing tools make it easier to test the application across different devices and configurations, reducing the time and effort required for testing and ensuring compatibility.
User Interface Design and User Experience Considerations

One of the biggest challenges in cross platform development is designing user interfaces that provide a consistent and seamless user experience across multiple platforms. With so many different devices and operating systems available, it can be difficult to ensure that an application looks and behaves in the same way on all of them.
One effective solution to this challenge is to use responsive design techniques that allow the user interface to adapt to different screen sizes and resolutions. This can involve creating multiple layouts or using a grid-based system that ensures that the interface elements are evenly spaced when displayed on different devices.
Usability Testing
In addition to responsive design, usability testing is also crucial to ensuring a positive user experience. Usability testing involves observing how users interact with the application and identifying any pain points or areas of confusion.
Usability testing can be conducted using a variety of methods, including user surveys, focus groups, and in-person testing sessions. Insights obtained from usability testing can be used to refine the user interface and improve the overall user experience.

Performance Optimization Techniques
Cross platform development can present many challenges, especially when it comes to optimizing the performance of applications. Resource management, memory allocation, and the hardware capabilities of different devices and operating systems all play a significant role in determining the overall performance of a cross platform application.
Developers must consider several factors when optimizing the performance of their cross platform applications. Here are some effective solutions:
- Code optimization: Optimizing code is critical to improving the performance of cross platform applications. Code can be optimized by removing redundant or unnecessary code, improving data structures, and using algorithms that require less processing time.
- Caching: Caching is an effective technique for improving the performance of cross platform applications. It reduces the amount of data that needs to be retrieved from a server, improving load times and reducing network usage.
- Resource management: Cross platform applications must manage resources effectively to ensure optimal performance. This includes managing memory, file storage, and network usage.
- Image compression: Images can significantly impact the performance of cross platform applications, especially on devices with limited resources. Compressing images can reduce their file size, decreasing load times and improving performance.
By implementing these effective solutions, developers can optimize the performance of their cross platform applications and ensure a smooth user experience across all devices and operating systems.
Code Reusability and Maintenance

In cross platform development, code reusability and maintenance present significant challenges that developers must address to ensure the smooth functioning of the application. These challenges stem from the differences in operating systems, software versions and hardware configurations of the devices that the application will run on. These differences may require platform-specific code modifications, adding complexity to the development process.
However, an effective solution to this challenge is to modularize the code and reduce platform-specific dependencies. This approach allows for greater code reusability and minimizes the need for platform-specific modifications, thereby simplifying the development process.
Modularizing Code

Modularizing code involves breaking down the application into smaller, independent modules, each with a particular functionality. This approach enables developers to focus on one section of the code at a time, making it easier to maintain and debug the code.
By using modularized code, developers can also reuse code across platforms, saving time and effort. For example, by using a single shared codebase, developers can easily create multiple versions of the application for different platforms, minimizing the need for platform-specific modifications.
Code Example
To illustrate the concept of modularizing code in the context of cross-platform development, let’s consider an example using Flutter, a popular cross-platform framework. In this example, we’ll break down a simple application into modular components, focusing on a scenario where we have a shared business logic module and separate UI modules for different platforms.
Step 1: Define a Shared Business Logic Module
First, we create a Dart file for our shared business logic, which can be reused across multiple platforms without modification.
lib/core/user_service.dart:
class UserService {
// Simulates fetching user data from a network or database
Future<String> fetchUserData() async {
// In a real app, this method would be asynchronous and fetch data from a server
return Future.delayed(Duration(seconds: 1), () => 'John Doe');
}
}
Step 2: Create Platform-specific UI Modules
Next, we’ll create platform-specific UI modules that utilize the shared business logic. While the business logic remains the same, each UI module can be tailored to fit the design guidelines and user experience of each platform.
lib/ui/mobile/user_screen_mobile.dart:
import 'package:flutter/material.dart';
import '../core/user_service.dart';
class UserScreenMobile extends StatelessWidget {
final UserService _userService = UserService();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('User Profile')),
body: FutureBuilder(
future: _userService.fetchUserData(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return CircularProgressIndicator();
} else {
return Center(child: Text('Welcome, ${snapshot.data}!'));
}
},
),
);
}
}
lib/ui/desktop/user_screen_desktop.dart:
import 'package:flutter/material.dart';
import '../core/user_service.dart';
class UserScreenDesktop extends StatelessWidget {
final UserService _userService = UserService();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('User Profile - Desktop')),
body: FutureBuilder(
future: _userService.fetchUserData(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return CircularProgressIndicator();
} else {
return Center(child: Text('Welcome to the desktop version, ${snapshot.data}!'));
}
},
),
);
}
}
Step 3: Main Application Entry
Finally, we determine which UI module to load based on the platform. This decision could be based on the screen size, operating system, or other factors.
lib/main.dart:
import 'package:flutter/material.dart';
import 'ui/mobile/user_screen_mobile.dart';
import 'ui/desktop/user_screen_desktop.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// Here we could decide which platform UI to present
// This is just a placeholder condition
bool isDesktop = MediaQuery.of(context).size.width > 600;
return MaterialApp(
home: isDesktop ? UserScreenDesktop() : UserScreenMobile(),
);
}
}
Explanation
In this modularized code example:
- The
UserService
class represents the shared business logic module, which can be reused across different platforms. - The
UserScreenMobile
andUserScreenDesktop
classes represent platform-specific UI modules that depend on the shared business logic. - The main application entry point decides which UI module to load based on certain conditions, such as screen size or platform type.
This approach demonstrates how modularizing code not only makes the codebase easier to manage and maintain but also facilitates code reuse across different platforms, aligning well with the principles of cross-platform development.

Minimizing Platform-Specific Dependencies
Minimizing platform-specific dependencies is another effective solution to code reusability and maintenance. This approach involves avoiding the use of platform-specific APIs, libraries, and frameworks, which can add complexity to the code and make it difficult to maintain.
Instead, developers can use cross platform development frameworks that provide abstraction layers for interacting with platform-specific APIs, making it easier to write code that works across multiple platforms. For example, frameworks like React Native, Xamarin, and Flutter provide developers with the tools they need to create cross platform applications without the need for platform-specific code modifications.
To overcome the challenges of code reusability and maintenance in cross platform development, developers must focus on modularizing the code and minimizing platform-specific dependencies. By doing so, they can create more maintainable and reusable code, simplifying the development process and enabling the creation of high-quality cross platform applications.
Cross Platform Testing and Debugging
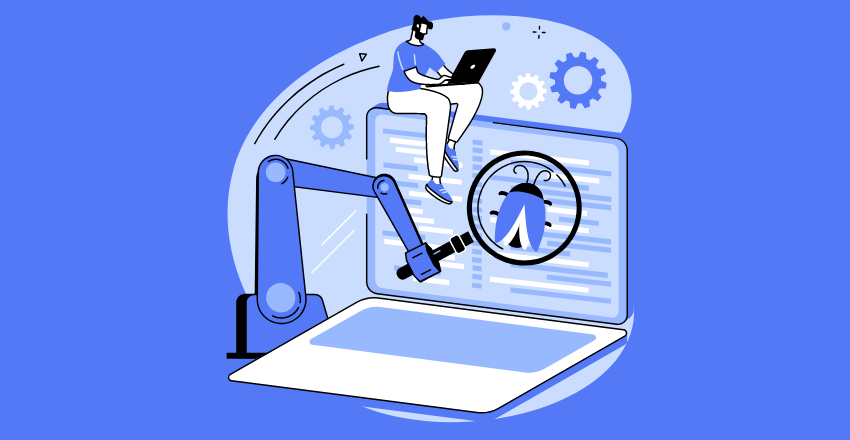
Testing and debugging are critical steps in the cross platform development process. Ensuring compatibility across multiple platforms and configurations can be challenging and time-consuming. However, by following best practices and utilizing effective tools, developers can streamline the testing and debugging process and ensure that their applications function seamlessly across all targeted platforms.
Cross Platform Testing Challenges
One of the biggest challenges in cross platform testing is the need to accommodate a wide range of devices, operating systems, and software versions. Each platform has its own unique characteristics and quirks that developers must consider when designing and testing an application. Compatibility issues, such as varying screen sizes and resolutions, can also create obstacles in the testing process.
Effective testing requires a carefully crafted plan that includes a range of testing methodologies and tools. Unit testing, integration testing, and acceptance testing are all important components of the testing process. In addition, automated testing tools can be a valuable asset for cross platform development teams, accelerating the testing process and eliminating the risk of human error.
Cross Platform Debugging Techniques
Debugging cross platform applications can be challenging due to the complexity of the development environment. Issues may arise due to platform-specific code or compatibility issues, making it difficult to pinpoint the root cause of a problem. Fortunately, there are a wide range of debugging techniques and tools available to help developers identify and resolve issues.
Remote debugging tools can be particularly useful in cross platform development, allowing developers to view and debug code on multiple platforms from a single machine. These tools allow developers to set breakpoints and analyze code in real-time, making it easier to locate and fix issues. In addition, logging and error reporting tools can be a valuable asset for identifying and tracking down issues, providing developers with detailed diagnostic information that can be used to resolve issues.
Effective Solutions for Cross Platform Testing and Debugging
There are several effective solutions that developers can implement to streamline the cross platform testing and debugging process. These include:
- Investing in automated testing tools to accelerate the testing process and reduce the risk of human error.
- Developing a comprehensive testing plan that includes unit testing, integration testing, and acceptance testing.
- Using remote debugging tools to view and debug code on multiple platforms from a single machine.
- Implementing logging and error reporting tools to provide detailed diagnostic information and track down issues.
- Conducting thorough testing on all targeted platforms, including a variety of devices, operating systems, and software versions, to ensure maximum compatibility.
By utilizing these effective solutions, developers can overcome the challenges of cross platform testing and debugging, ensuring that their applications are optimized for performance and functionality across all targeted platforms.
Security and Privacy Concerns

Cross platform development brings about unique security and privacy challenges that require careful consideration during the development process. These challenges include:
- Data encryption: Sensitive data should be encrypted to prevent unauthorized access. Developers should use reliable encryption algorithms to safeguard data on both client and server sides.
- Secure communications: Communication channels should be secured to prevent interception and tampering. Developers should use SSL/TLS protocols to ensure secure data exchange between applications and servers.
- App permissions: Cross platform apps often require access to a device’s sensitive data or features, such as location data or camera access. Developers must ensure that app permissions are used appropriately and that apps do not access device features unnecessarily.
Dealing with development obstacles like these requires a collaborative approach between developers, designers, and security experts. It is important to follow industry best practices and adhere to security standards to ensure the safety and privacy of users’ data.

Collaborative Development and Communication
Cross platform development often requires collaboration among geographically dispersed teams. This can lead to communication challenges that can hinder the development process. To overcome this obstacle, it is essential to establish clear communication channels and implement collaboration tools that facilitate efficient and effective teamwork.
One effective communication strategy is to define clear project goals and objectives, establish a common vocabulary and terminology, and clearly define roles and responsibilities for each team member. This clarity can ensure that all team members are aligned and working towards the same objectives.
Another effective strategy is to use collaboration tools such as version control systems and issue trackers. These tools can help streamline project management and improve communication among team members. Popular tools include Git, Jira, and Trello.
Regular team meetings can also help improve communication and collaboration. These meetings can be conducted via video conferencing platforms and should focus on updating team members on project progress, discussing challenges and solutions, and identifying any potential roadblocks or risks that may impact project completion.
Effective communication and collaboration are critical to successful cross platform development. By utilizing the strategies and tools outlined above, teams can overcome communication challenges and work together efficiently and effectively towards project completion.
Third-Party Integration and APIs

Integrating third-party services and APIs into cross-platform applications can present a unique set of challenges. Dealing with development obstacles such as compatibility issues, varying data formats, authentication, and security concerns can all make the process of integrating APIs more complex. However, there are some effective solutions that can help developers navigate these challenges.
When selecting a third-party service or API, it is important to consider how it will integrate with the different platforms you are targeting. You should also choose services that provide clear documentation, support, and have a proven track record of cross-platform compatibility. Developers should take advantage of SDKs and libraries that simplify the integration process.
It is also important to ensure that the API is secure and that any user data is protected. Developers must carefully consider the access control and permissions that will be granted to the third-party service. They should also make sure that any data transmitted to and from the API is encrypted and that secure communication protocols are used.
When it comes to compatibility issues, developers should test the API on different platforms and devices to ensure that it works seamlessly across all targeted platforms. This is especially important when dealing with APIs that rely on specific device sensors or hardware features.
Continuous Integration and Deployment
Cross platform application development involves creating software that operates seamlessly across multiple platforms. However, continuous integration and deployment can be a significant challenge in such projects. Developers must ensure that code changes on one platform do not cause issues on other platforms. They must also ensure that new features are rolled out consistently across all platforms. Additionally, app updates must be released simultaneously across different devices and operating systems.
One effective solution to these challenges is to use continuous integration and deployment (CI/CD) pipelines. CI/CD pipelines automate the build, testing, and release processes, making it easier to maintain consistency across platforms. By utilizing CI/CD pipelines, developers can easily identify and resolve issues on different platforms and ensure that new features are deployed quickly and seamlessly.
Benefits of CI/CD for Cross Platform Development
CI/CD pipelines offer numerous benefits for cross platform development projects, including:
- Increased efficiency and productivity
- Improved code quality and consistency
- Reduced development and deployment time
- Elimination of human error in the build and deployment processes
- Improved team collaboration and communication
CI/CD Tools for Cross Platform Development
Multiple CI/CD tools are available for cross platform development, including Jenkins, Travis CI, and CircleCI. Such tools support multiple programming languages, frameworks, and platforms and offer integrations with popular version control systems like Git.
Implementing a CI/CD pipeline requires a dedicated workflow and testing strategy for each platform. The pipeline must include automated testing, continuous integration, and deployment to ensure the smooth functioning of cross platform applications.
Utilizing a CI/CD pipeline can significantly improve the efficiency and quality of cross platform development. By identifying and resolving issues early in the development process, developers can avoid delays and ensure that updates are deployed consistently across all platforms.
Cross Platform Development Frameworks
Cross platform development frameworks provide developers with the tools to create applications that run smoothly across multiple platforms, without the need for platform-specific modifications. They can help mitigate many of the challenges associated with cross platform development, including compatibility issues, code reusability, and user interface design.
React Native

React Native is a popular cross platform development framework for building native iOS and Android applications using JavaScript and React. It allows developers to write code once and deploy it to multiple platforms, reducing development time and costs. React Native also provides a range of pre-built components that make it easy to design visually appealing user interfaces.
Pros | Cons |
---|---|
Large and active community | May require additional modules to access certain mobile features |
Quick and easy development process | Less customizable than other frameworks |
Smooth performance and responsiveness | Limited debugging tools |
Xamarin
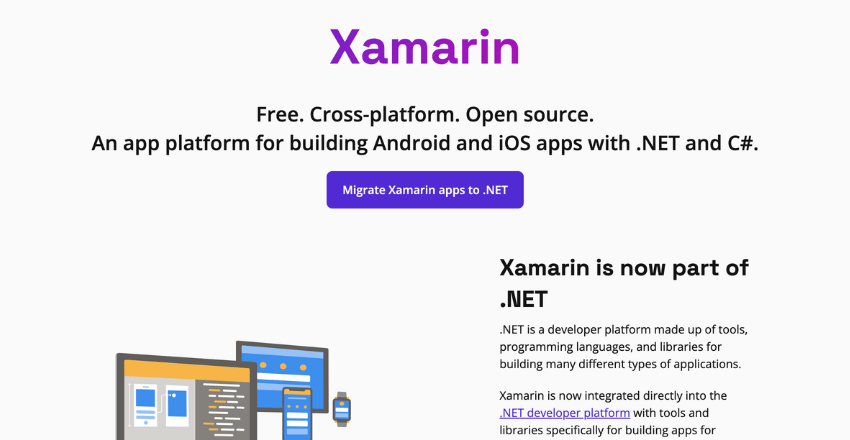
Xamarin is a cross platform development framework that allows developers to build native applications using C# and .NET. It provides access to native API’s and platform-specific features, allowing for greater customization and flexibility in the development process. Xamarin also includes a range of built-in testing and debugging tools to simplify the development process.
Pros | Cons |
---|---|
Access to native API’s and platform-specific features | Less support for third-party plugins than other frameworks |
Powerful and flexible development environment | Requires knowledge of C# and .NET |
Integrated testing and debugging tools | Less community support than other frameworks |
Flutter

Flutter is a relatively new cross platform development framework that allows developers to create high-performance, visually stunning applications for iOS, Android, and other platforms using a single codebase. It uses Dart, a modern object-oriented programming language, and includes a range of pre-built widgets and libraries that make it easy to design custom user interfaces.
Pros | Cons |
---|---|
High-performance and visually stunning applications | Less community support than other frameworks |
Quick and easy development process | Requires knowledge of Dart programming language |
Hot reload feature allows for quick iteration and testing | Less support for third-party plugins than other frameworks |
Overall, cross platform development frameworks can provide valuable solutions to the challenges associated with developing applications for multiple platforms. By understanding the unique features and benefits of each framework, developers can select the best tool for their needs and create high-quality, cross-platform applications that meet their users’ needs.
Best Practices for Cross Platform Development
Developing cross platform applications is challenging, but implementing effective solutions can help overcome these obstacles. Here are some best practices for successful cross platform development:
- Organize your code: Keeping your code organized and modularized can help reduce complexity and make it easier to maintain and update over time.
- Document your code: Documenting your code is essential for maintaining clear communication and ensuring that others can understand and modify your code in the future.
- Use version control: Version control is critical for managing code changes and coordinating development efforts across teams.
- Focus on user experience: Putting user experience first is key to ensuring that your application is successful. Make sure to design interfaces that are intuitive and responsive on all platforms.
- Test rigorously: Testing your application across all platforms and devices is essential for identifying and resolving compatibility issues.
- Stay up-to-date: Keeping up-to-date with platform-specific updates and releases can help ensure that your application remains relevant and compatible over time.
By following these best practices, developers can create cross platform applications that are both functional and user-friendly.
Examples of Cross Platform Applications
Example 1: Airbnb

Airbnb is a popular online marketplace that connects travelers with hosts for lodging and experiences. The company faced the challenge of delivering a seamless user experience across different platforms and devices. To overcome this obstacle, Airbnb used React Native, a popular cross platform development framework. This allowed them to write code once and deploy it across multiple platforms, resulting in a consistent user experience and faster development time.
Example 2: Uber
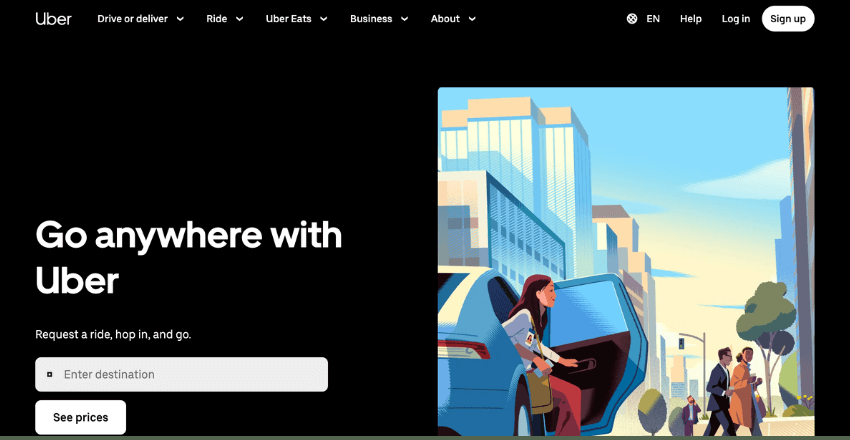
Uber, a leading ride-sharing service, needed to develop and deploy their app quickly across multiple platforms to keep up with their growing demand. They leveraged the Xamarin platform, which allowed them to write code in C# and deploy it across iOS, Android, and Windows devices. As a result, they were able to quickly release a high-quality app that provided a seamless experience to their users.
Example 3: Walmart

Walmart, one of the largest retailers in the world, faced the challenge of providing a consistent experience for their customers across different platforms and devices. They chose to use Flutter, a cross platform development framework, to address this issue. By leveraging Flutter’s hot reload feature, Walmart was able to significantly reduce development time and provide a consistent experience to their customers on both iOS and Android platforms.
These examples demonstrate the importance of finding effective solutions to the challenges faced in cross platform development. By utilizing the right tools and frameworks, companies can achieve success and provide a seamless experience to their users.
Wrapping up
Cross platform development comes with numerous challenges that can hinder the development process. However, with effective solutions, it is possible to overcome these challenges and create successful cross platform applications.
External Resources
https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Client-side_web_APIs/Third_party_APIs
FAQ

1. How do you manage state efficiently across multiple platforms?
Challenge: State management is crucial for maintaining app performance and user experience. Cross-platform frameworks offer their solutions, but inconsistencies in state management across platforms can lead to bugs and poor performance.
Answer: Leveraging platform-agnostic state management libraries can help. For example, using Redux in React Native or Provider and Riverpod in Flutter ensures a consistent approach to state management across iOS and Android.
React Native Redux Example:
import { createStore } from 'redux';
// Reducer function
function counter(state = 0, action) {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
}
// Create Redux store
const store = createStore(counter);
// Usage: Dispatch actions to update state
store.dispatch({ type: 'INCREMENT' });
console.log(store.getState()); // Output: 1
Explanation: This example shows a basic Redux setup in React Native, where a store is created with a simple reducer function to manage state changes based on actions. Redux provides a predictable state container across platforms.
2. How do you ensure a consistent user interface (UI) across different platforms?
Challenge: Each platform has its design language (e.g., Material Design on Android and Human Interface Guidelines on iOS). Achieving UI consistency while respecting platform conventions can be difficult.
Answer: Utilize cross-platform UI libraries that offer a cohesive look and feel while allowing for platform-specific customizations. For instance, Flutter’s widget system or React Native’s platform-specific style sheets can achieve this balance.
Flutter Platform-Specific Widgets Example:
import 'package:flutter/foundation.dart' show kIsWeb;
import 'package:flutter/material.dart';
Widget buildPlatformSpecificWidget() {
if (kIsWeb) {
return Text('Running on the web');
} else {
return MaterialApp(
home: Scaffold(
body: Center(
child: kIsWeb ? Text('Web view') : Text('Mobile view'),
),
),
);
}
}
Explanation: This Flutter code snippet demonstrates how to use conditional statements to render platform-specific widgets, ensuring that the app fits well within the design ethos of each platform while maintaining overall UI consistency.
3. What strategies can be employed to handle platform-specific functionalities?
Challenge: Certain features are inherently platform-specific, such as push notifications, deep linking, or accessing device hardware (camera, GPS). Implementing these features in a cross-platform app can be challenging.
Answer: Use platform channels in Flutter or Native Modules in React Native to communicate between the cross-platform code and the platform-specific native code. This allows developers to implement platform-specific functionalities when necessary.
Flutter Platform Channel Example:
import 'package:flutter/services.dart';
class NativeCodeRunner {
static const platform = MethodChannel('com.example/native');
Future<void> runNativeCode() async {
try {
final String result = await platform.invokeMethod('runNative');
print(result);
} on PlatformException catch (e) {
print("Failed to run native code: '${e.message}'.");
}
}
}
Explanation: This Flutter example uses a method channel to invoke a method on the native side (runNative
). This approach enables the execution of platform-specific code (e.g., accessing a hardware feature) that cannot be directly accessed through the cross-platform framework.

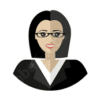
Charlotte Williams is a talented technical author specializing in cross-platform app development. With a diverse professional background, she has gained valuable experience at renowned companies such as Alibaba and Accenture. Charlotte’s journey in the tech industry began as a mobile UX designer back in 2007, allowing her to develop a keen understanding of user-centric app design.
Proficient in utilizing frameworks like React Native and Flutter, Charlotte excels in building cross-platform mobile apps and imparting her knowledge to aspiring developers. She pursued a degree in Computer Science at Cornell University, equipping her with a strong foundation in the field. Residing in San Francisco with her three beloved dogs, she finds solace in hiking the hills and connecting with nature. Charlotte’s passion for app development, combined with her dedication to sharing expertise, makes her an invaluable resource in the world of cross-platform app development.